Cloud Storage Access through API - ASP.NET MVC 5 - C# (Part-7)
Google Picker API - File Open dialog Box,
File Uploading,
File View & Sharing,
File Downloading,
2) Google Drive API - File Upload, View, Download & Delete
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
Google Picker API - File Open dialog Box,
File Uploading,
File View & Sharing,
File Downloading,
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
In this tutorial we are learn:
◘ What is Google Picker API?
◘ Why use Google Picker API?
◘ How to Enable & Register Google Picker API for your application?
◘ How to Create Google Picker API Project using Java Script?
◘ Discuss some important features of Google Picker API.
◘ A Real Life Example of Google Picker API.
A) What is Google Picker API?
Google Picker is a "File Open" dialog box for the information stored in Google Servers. With Google Picker, we can access and upload photos, videos, maps and documents stored in Google servers. We use this interface to our web page or web application.
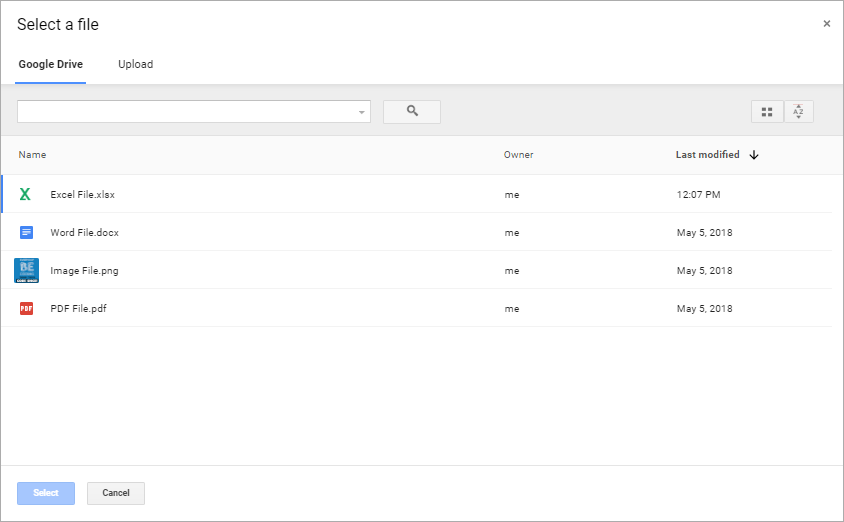
B) Why use Google Picker API?
◘ Google Picker is just like a Graphical interface of Google drive and other Google properties.
◘ It providing users a more modern experience. A dialog experience with many views showing previews or thumbnails and many more.
◘ Web developers can incorporate Google Picker API by just adding a few lines of Java Scripts code.
Mainly Google Picker API is use for View, Open, Upload & Searching file information form
◘ Google Drive Items,
◘ Google Picasa Web Photo albums.
◘ YouTube videos.
◘ Google Map.
◘ Google Image, Video Searching & many more...
◘ Goto this Link: https://console.developers.google.com/flows/enableapi?apiid=picker&credential=client_key.
Which guides you through create a project in the Google Picker API Console for enabling API & creating credentials.
◘ If you haven't done so already, create your application's API key by clicking Create Credentials -> API Key. Next Look for your API key in the API key section.
◘ If you haven't done so already, create your OAuth 2.0 credentials by clicking Create Credentials -> OAuth Client ID. After your are created the credentials, you can see your client ID on the credentials page. Click the client ID for details, such as client secret, redirect URLs, Java Script origins address and email address.
D): Create Google Picker API Project using Java Script
There are few simple steps required to create a Google Picker API project.
◘ First, use Visual Studio 2013 or Higher Version & Create an Empty ASP.NET MVC Project. Set "GooglePickerAPI" as a name of your Project.
Add a controller class HomeController.cs under this controller folder.
Add new Index action method & add view of this action index action method.
◘ Second, Just copy & paste this below code into the view (Index.cshtml).
<center>
<h2>Google Picker API</h2>
<table class="imagetable">
<tbody>
<tr id="header">
<th style="font-size:18px;">Google Drive</th>
</tr>
<tr>
<td style="font-size:16px;"><a href="#" id="AllFilePick">All Drive items.</a></td>
</tr>
</tbody>
</table>
</center>
<script type="text/javascript">
//=Create object of FilePicker Constructor function function & set Properties===
function SetPicker() {
var picker = new FilePicker(
{
apiKey: 'XXXXXXXXXXXXXXX',
clientId: 'XXXXXXXXX-XXXXXXXXXXXXX.apps.googleusercontent.com',
buttonEl: document.getElementById("AllFilePick"),
onClick: function (file) {
PopupCenter('https://drive.google.com/file/d/' + file.id + '/view', "", 1026, 500);
}});
}
//====================Create POPUP function==============
function PopupCenter(url, title, w, h)
{
var left = (screen.width / 2) - (w / 2);
var top = (screen.height / 2) - (h / 2);
return window.open(url, title, 'width=' + w + ', height=' + h + ', top=' + top + ', left=' + left); }
//===============Create Constructor function==============
function FilePicker(User)
{
//Configuration
this.apiKey = User.apiKey;
this.clientId = User.clientId;
//Button
this.buttonEl = User.buttonEl;
//Click Events
this.onClick = User.onClick;
this.buttonEl.addEventListener('click', this.open.bind(this));
//Disable the button until the API loads, as it won't work properly until then.
this.buttonEl.disabled = true;
//Load the drive API
gapi.client.setApiKey(this.apiKey);
gapi.client.load('drive', 'v2', this.DriveApiLoaded.bind(this));
gapi.load('picker', '1', { callback: this.PickerApiLoaded.bind(this) });
}
FilePicker.prototype = {
//==========Check Authentication & Call ShowPicker() function=======
open: function () {
// Check if the user has already authenticated
var token = gapi.auth.getToken();
if (token)
{
this.ShowPicker();
} else {
// The user has not yet authenticated with Google
// We need to do the authentication before displaying the drive picker.
this.DoAuth(false, function ()
{ this.ShowPicker(); }.bind(this));
}
},
//========Show the file picker once authentication has been done.=========
ShowPicker: function () {
var accessToken = gapi.auth.getToken().access_token;
//========Show Different Display View in Picker Dialog box=======
//View all the documents of Google drive
//var DisplayView = new google.picker.View(google.picker.ViewId.DOCS);
//View all the documents of a Specific folder of Google drive
//var DisplayView = new google.picker.DocsView().setParent('PUT YOUR FOLDER ID');
//View all the documents & folders of google drive
var DisplayView = new google.picker.DocsView().setIncludeFolders(true);
//Only view all Folders in Google drive.
//var DisplayView = new google.picker.DocsView()
// .setIncludeFolders(true)
// .setMimeTypes('application/vnd.google-apps.folder')
// .setSelectFolderEnabled(true);
//Use DocsUploadView to upload documents to Google Drive.
//var UploadView = new google.picker.DocsUploadView();
//addViewGroup(new google.picker.ViewGroup(google.picker.ViewId.DOCS).
// addView(google.picker.ViewId.DOCUMENTS).
// addView(google.picker.ViewId.PRESENTATIONS)).
//========Show Different Upload View in Picker Dialog box=======
//User can upload file in any folder (by select folder)
var UploadView = new google.picker.DocsUploadView().setIncludeFolders(true);
//User can upload file in specific folder
//var UploadView = new google.picker.DocsUploadView().setParent('PUT YOUR FOLDER ID')
this.picker = new google.picker.PickerBuilder().
addView(DisplayView).
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
setAppId(this.clientId).
addView(UploadView).
setOAuthToken(accessToken).
setCallback(this.PickerResponse.bind(this)).
setTitle('Google Picker API - EVERYDAY BE CODING').
build().
setVisible(true);
},
//====Called when a file has been selected in the Google Picker Dialog Box===
PickerResponse: function (data) {
if (data[google.picker.Response.ACTION] == google.picker.Action.PICKED) {
var file = data[google.picker.Response.DOCUMENTS][0],
id = file[google.picker.Document.ID],
request = gapi.client.drive.files.get({ fileId: id });
this.ShowPicker();
request.execute(this.GetFileDetails.bind(this));
}
},
//====Called when file details have been retrieved from Google Drive.========
GetFileDetails: function (file) {
if (this.onClick) {
this.onClick(file);
}
},
//====Called when the Google Drive file picker API has finished loading.=======
PickerApiLoaded: function () {
this.buttonEl.disabled = false;
},
//========Called when the Google Drive API has finished loading.==========
DriveApiLoaded: function () {
this.DoAuth(true);
},
//========Authenticate with Google Drive via the Google Picker API.=====
DoAuth: function (immediate, callback) {
gapi.auth.authorize({
client_id: this.clientId,
scope: 'https://www.googleapis.com/auth/drive',
immediate: immediate
}, callback);
}
};
</script>
<script src="https://www.google.com/jsapi?key=<YOUR API KEY>"></script>
<script src="https://apis.google.com/js/client.js?onload=SetPicker"></script>
E) Discuss some important features of Google Picker API
By using Google Google Picker API we can access documents in advanced way,
The main concept is that create a Picker object using PickerBuilder() constructor function. The Picker instance represents the Google Picker Dialog Box & this Picker Dialog box rendered on web page inside a Iframe.
Source Code:
this.picker = new google.picker.PickerBuilder().
addView(new google.picker.DocsView().setIncludeFolders(true)).
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
setAppId(this.clientId).
addView(new google.picker.DocsUploadView().setIncludeFolders(true)).
setOAuthToken(accessToken).
setCallback(this.PickerResponse.bind(this)).
setTitle('Google Picker API - EVERYDAY BE CODING').
build().
setVisible(true);
For example there are some basic important features are
◘ Not only for view, we can upload file using Google Picker Dialog Box.
addView(new google.picker.DocsUploadView().setIncludeFolders(true))
◘ We can select & Upload single or multiple file at a time using Google Picker API.
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
◘ We can Upload file into the folders by select which folder you wants.
addView(new google.picker.DocsUploadView().setIncludeFolders(true))
◘ We can filter your view as per your requirements.
◘ For all Documents & Folders:
addView(new google.picker.DocsView().setIncludeFolders(true))
◘ Only for Documents:
addView(new google.picker.View(google.picker.ViewId.DOCS))
◘ Only for Presentation Documents:
addView(new google.picker.View(google.picker.ViewId.PRESENTATIONS))
◘ Only for Spreadsheet Documents:
addView(new google.picker.View(google.picker.ViewId.SPREADSHEETS))
◘ Only for Images:
addView(new google.picker.View(google.picker.ViewId.DOCS_IMAGES))
if You have more queries about this Google Picker API,
Please visit this Link: https://developers.google.com/picker/docs/
E) A Real Life Example of Google Picker API
A real life application development is depends on our client requirements. Suppose a company has three departments and each of those departments has difference documents structures.
For Example HR Departments has documents, those are only accessible for HR employees & it is fully restricted for others departments & Account Departments documents are only for account employees & as well as IT Departments documents are only for IT Employees.
Which guides you through create a project in the Google Picker API Console for enabling API & creating credentials.
◘ If you haven't done so already, create your application's API key by clicking Create Credentials -> API Key. Next Look for your API key in the API key section.
◘ If you haven't done so already, create your OAuth 2.0 credentials by clicking Create Credentials -> OAuth Client ID. After your are created the credentials, you can see your client ID on the credentials page. Click the client ID for details, such as client secret, redirect URLs, Java Script origins address and email address.
D): Create Google Picker API Project using Java Script
There are few simple steps required to create a Google Picker API project.
◘ First, use Visual Studio 2013 or Higher Version & Create an Empty ASP.NET MVC Project. Set "GooglePickerAPI" as a name of your Project.
Add new Index action method & add view of this action index action method.
◘ Second, Just copy & paste this below code into the view (Index.cshtml).
<center>
<h2>Google Picker API</h2>
<table class="imagetable">
<tbody>
<tr id="header">
<th style="font-size:18px;">Google Drive</th>
</tr>
<tr>
<td style="font-size:16px;"><a href="#" id="AllFilePick">All Drive items.</a></td>
</tr>
</tbody>
</table>
</center>
<script type="text/javascript">
//=Create object of FilePicker Constructor function function & set Properties===
function SetPicker() {
var picker = new FilePicker(
{
apiKey: 'XXXXXXXXXXXXXXX',
clientId: 'XXXXXXXXX-XXXXXXXXXXXXX.apps.googleusercontent.com',
buttonEl: document.getElementById("AllFilePick"),
onClick: function (file) {
PopupCenter('https://drive.google.com/file/d/' + file.id + '/view', "", 1026, 500);
}});
}
//====================Create POPUP function==============
function PopupCenter(url, title, w, h)
{
var left = (screen.width / 2) - (w / 2);
var top = (screen.height / 2) - (h / 2);
return window.open(url, title, 'width=' + w + ', height=' + h + ', top=' + top + ', left=' + left); }
//===============Create Constructor function==============
function FilePicker(User)
{
//Configuration
this.apiKey = User.apiKey;
this.clientId = User.clientId;
//Button
this.buttonEl = User.buttonEl;
//Click Events
this.onClick = User.onClick;
this.buttonEl.addEventListener('click', this.open.bind(this));
//Disable the button until the API loads, as it won't work properly until then.
this.buttonEl.disabled = true;
//Load the drive API
gapi.client.setApiKey(this.apiKey);
gapi.client.load('drive', 'v2', this.DriveApiLoaded.bind(this));
gapi.load('picker', '1', { callback: this.PickerApiLoaded.bind(this) });
}
FilePicker.prototype = {
//==========Check Authentication & Call ShowPicker() function=======
open: function () {
// Check if the user has already authenticated
var token = gapi.auth.getToken();
if (token)
{
this.ShowPicker();
} else {
// The user has not yet authenticated with Google
// We need to do the authentication before displaying the drive picker.
this.DoAuth(false, function ()
{ this.ShowPicker(); }.bind(this));
}
},
//========Show the file picker once authentication has been done.=========
ShowPicker: function () {
var accessToken = gapi.auth.getToken().access_token;
//========Show Different Display View in Picker Dialog box=======
//View all the documents of Google drive
//var DisplayView = new google.picker.View(google.picker.ViewId.DOCS);
//View all the documents of a Specific folder of Google drive
//var DisplayView = new google.picker.DocsView().setParent('PUT YOUR FOLDER ID');
//View all the documents & folders of google drive
var DisplayView = new google.picker.DocsView().setIncludeFolders(true);
//Only view all Folders in Google drive.
//var DisplayView = new google.picker.DocsView()
// .setIncludeFolders(true)
// .setMimeTypes('application/vnd.google-apps.folder')
// .setSelectFolderEnabled(true);
//Use DocsUploadView to upload documents to Google Drive.
//var UploadView = new google.picker.DocsUploadView();
//addViewGroup(new google.picker.ViewGroup(google.picker.ViewId.DOCS).
// addView(google.picker.ViewId.DOCUMENTS).
// addView(google.picker.ViewId.PRESENTATIONS)).
//========Show Different Upload View in Picker Dialog box=======
//User can upload file in any folder (by select folder)
var UploadView = new google.picker.DocsUploadView().setIncludeFolders(true);
//User can upload file in specific folder
//var UploadView = new google.picker.DocsUploadView().setParent('PUT YOUR FOLDER ID')
this.picker = new google.picker.PickerBuilder().
addView(DisplayView).
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
setAppId(this.clientId).
addView(UploadView).
setOAuthToken(accessToken).
setCallback(this.PickerResponse.bind(this)).
setTitle('Google Picker API - EVERYDAY BE CODING').
build().
setVisible(true);
},
//====Called when a file has been selected in the Google Picker Dialog Box===
PickerResponse: function (data) {
if (data[google.picker.Response.ACTION] == google.picker.Action.PICKED) {
var file = data[google.picker.Response.DOCUMENTS][0],
id = file[google.picker.Document.ID],
request = gapi.client.drive.files.get({ fileId: id });
this.ShowPicker();
request.execute(this.GetFileDetails.bind(this));
}
},
//====Called when file details have been retrieved from Google Drive.========
GetFileDetails: function (file) {
if (this.onClick) {
this.onClick(file);
}
},
//====Called when the Google Drive file picker API has finished loading.=======
PickerApiLoaded: function () {
this.buttonEl.disabled = false;
},
//========Called when the Google Drive API has finished loading.==========
DriveApiLoaded: function () {
this.DoAuth(true);
},
//========Authenticate with Google Drive via the Google Picker API.=====
DoAuth: function (immediate, callback) {
gapi.auth.authorize({
client_id: this.clientId,
scope: 'https://www.googleapis.com/auth/drive',
immediate: immediate
}, callback);
}
};
</script>
<script src="https://www.google.com/jsapi?key=<YOUR API KEY>"></script>
<script src="https://apis.google.com/js/client.js?onload=SetPicker"></script>
E) Discuss some important features of Google Picker API
By using Google Google Picker API we can access documents in advanced way,
The main concept is that create a Picker object using PickerBuilder() constructor function. The Picker instance represents the Google Picker Dialog Box & this Picker Dialog box rendered on web page inside a Iframe.
Source Code:
this.picker = new google.picker.PickerBuilder().
addView(new google.picker.DocsView().setIncludeFolders(true)).
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
setAppId(this.clientId).
addView(new google.picker.DocsUploadView().setIncludeFolders(true)).
setOAuthToken(accessToken).
setCallback(this.PickerResponse.bind(this)).
setTitle('Google Picker API - EVERYDAY BE CODING').
build().
setVisible(true);
For example there are some basic important features are
◘ Not only for view, we can upload file using Google Picker Dialog Box.
addView(new google.picker.DocsUploadView().setIncludeFolders(true))
◘ We can select & Upload single or multiple file at a time using Google Picker API.
enableFeature(google.picker.Feature.MULTISELECT_ENABLED).
◘ We can Upload file into the folders by select which folder you wants.
addView(new google.picker.DocsUploadView().setIncludeFolders(true))
◘ We can filter your view as per your requirements.
◘ For all Documents & Folders:
addView(new google.picker.DocsView().setIncludeFolders(true))
◘ Only for Documents:
addView(new google.picker.View(google.picker.ViewId.DOCS))
◘ Only for Presentation Documents:
addView(new google.picker.View(google.picker.ViewId.PRESENTATIONS))
◘ Only for Spreadsheet Documents:
addView(new google.picker.View(google.picker.ViewId.SPREADSHEETS))
◘ Only for Images:
addView(new google.picker.View(google.picker.ViewId.DOCS_IMAGES))
if You have more queries about this Google Picker API,
Please visit this Link: https://developers.google.com/picker/docs/
E) A Real Life Example of Google Picker API
A real life application development is depends on our client requirements. Suppose a company has three departments and each of those departments has difference documents structures.
For Example HR Departments has documents, those are only accessible for HR employees & it is fully restricted for others departments & Account Departments documents are only for account employees & as well as IT Departments documents are only for IT Employees.
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
Please subscribe my YouTube Channel & don't forget to like and share.
YouTube : | https://goo.gl/rt4tHH |
Facebook : | https://goo.gl/hgpQsh |
Twitter : | https://goo.gl/nUwGnf |
Can this be implemented using NODEjs?? do you have any post related to nodeJS for Google picker api?
ReplyDelete