Cloud Storage Access through API - ASP.NET MVC 5 - C# (Part-5)
Google Drive REST API v3-
a) Create a folder with Share Permission.
b) How to Share & Set User Role Permission on Existing Files & Folders in Google Drive.
2) Google Drive API - File Upload, View, Download & Delete
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
Google Drive REST API v3-
a) Create a folder with Share Permission.
b) How to Share & Set User Role Permission on Existing Files & Folders in Google Drive.
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
Source Code: Click to download [11.1 MB]
This tutorial is a continuation of Part-1, Part-2, Part-3 & Part-4
In Part-4 of this video series, we have seen "Move & Copy files between folders in Google drive using Google drive API v3".
First of all, Cloud sharing or file sharing means you can set the permission of a file to access outside world in the network. for example, within a single user or a group of users or team.
If you want to share a files or folder in google drive, you need to set the permission of file & folders at the time of uploading or after uploading a file in google drive.
Programmatic we can Insert, Update, Delete drive item permission to file/folder in google drive using Google Drive Rest API in v3.
In this video, I will discuss only Insert drive item permission to file/folder in google drive.
In my upcoming tutorials, I will discuss "how to Update, Delete drive item permission to file/folders"
Programmatically permission can effect in mainly four property of drive API v3 Permission class
1) User Type
2) User Role
3) EmailAddress
4) Domain
1) User Type is classified as four type:
a) user - email address - for example everydaybecoding@gmail.com
b) group - group email address - for example GroupName@googlegroup.com
c) domain - domain name of G-Suit - for example thecompany.com
d) anyone -N/A - no permission is requird.
What is Google Group?
Google Groups is a group of people. such as project teams, departments & classmates. we can send an email to everyone in a group with one address, invite a group to an event, or share documents with a group.
2) User Role is classified as four type:
a) reader - only read the content, metadata of file & folder (like - name & description).
b) writer - all type of permission but not allow for delete file & folders.
c) owner - almost all type of permission but not allowed in Team Drives.
d) organizer - all type of Role is only valid for Google Team Drives.
For more Information about file ownership, please visit this link:
https://support.google.com/drive/answer/2494892?co=GENIE.Platform%3DDesktop&hl=en
What is Google Team Drive?
Google Team Drives are shared spaces where teams can easily store, search, and access their files anywhere, from any device.
Unlike files in My Drive, files in Team Drive belong to the team instead of an individual. Even if members leave, the files stay exactly where they are so your team can continue to share information and get work done.
For More Information visit this link:
https://gsuite.google.com/learning-center/products/drive/get-started-team-drive/
3) EmailAddress : Email address of User & Google groups. This property is present when permission type is user & groups.
4) Domain: Use any Google domain address, For Example, admin@company.com.
For More Information visit this link:
https://developers.google.com/drive/v3/web/about-permissions
A) Create a folder with Share Permission.
Please see this code:
public static string CreateFolderWithPermission(string FolderName, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
//Create Folder in Google Drive
Google.Apis.Drive.v3.DriveService service = GetService_v3();
var fileMetadata = new Google.Apis.Drive.v3.Data.File()
{
Name = FolderName,
MimeType = "application/vnd.google-apps.folder",
Description = "File_" + System.DateTime.Now.Hour + ":" + System.DateTime.Now.Minute + ":" + System.DateTime.Now.Second
};
Google.Apis.Drive.v3.FilesResource.CreateRequest request = service.Files.Create(fileMetadata);
request.Fields = "id";
string Fileid = request.Execute().Id;
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
permission = service.Permissions.Create(permission, Fileid).Execute();
}
else if (UserRole == "owner")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, Fileid);
createRequestPermission.TransferOwnership = true;
createRequestPermission.Execute();
}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
B) Enable Share & Set Permission to Google File/Folder.
As a 'reader','writer' you can share any file that you want, but in case of 'owner', you can only transfer ownership of Google files or Google items.
These are Google Docs, Google Sheets, Google Slides, Google Forms, Google Drawing, Google My Maps & Folders.
Please see this code:
public static string FileSharePermission(string fileId, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user"; //'user', 'group', 'domain', 'anyone' - all are small letter keyword
permission.EmailAddress = EmailAddress; // 'reader', 'writer', 'owner', 'organizer' - all are small letter keyword
permission.Role = UserRole; // use any Google account: emails or groups
permission = service.Permissions.Create(permission, fileId).Execute();
}
//else if (UserRole == "owner")
//{
// permission.Type = "user";
// permission.EmailAddress = EmailAddress;
// permission.Role = UserRole;
// Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, fileId);
// createRequestPermission.TransferOwnership = true;
// createRequestPermission.Execute();
//}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
Let's do it using Visual Studio MVC Application:
First, Goto the Visual Studio 2013 or Higher Version.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following command.
PM> Install-Package Google.Apis.Drive.v3
Here I am using Google Drive API v3,
Step 3 (Model):
First, we need to create one model class under the Model folder of project solution.
Model Class Name: GoogleDriveFiles.cs
Please Copy & Paste this code into this class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public IList<string> Parents { get; set; }
}
}
Step 4 (Repository):
After that, create another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Please Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFilesRepository
{
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//Create Drive API service.
Google.Apis.Drive.v3.DriveService service = new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(createdTime, id, name, size, version, trashed, parents, webViewLink)";
// Request of List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents,
};
FileList.Add(File);
}
}
return FileList;
}
public static void FileUpload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
string path = Path.Combine(HttpContext.Current.Server.MapPath("~/GoogleDriveFiles"),
Path.GetFileName(file.FileName));
file.SaveAs(path);
Google.Apis.Drive.v3.Data.File FileMetaData = new Google.Apis.Drive.v3.Data.File();
FileMetaData.Name = Path.GetFileName(file.FileName);
FileMetaData.MimeType = MimeMapping.GetMimeMapping(path);
Google.Apis.Drive.v3.FilesResource.CreateMediaUpload request;
using (var stream = new System.IO.FileStream(path, System.IO.FileMode.Open))
{
request = service.Files.Create(FileMetaData, stream, FileMetaData.MimeType);
request.Fields = "id";
request.Upload();
}
}
}
public static string FileSharePermission(string fileId, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user"; //'user', 'group', 'domain', 'anyone' - all are small letter keyword
permission.EmailAddress = EmailAddress; // 'reader', 'writer', 'owner', 'organizer' - all are small letter keyword
permission.Role = UserRole; // use any Google account: emails or groups
permission = service.Permissions.Create(permission, fileId).Execute();
}
//else if (UserRole == "owner")
//{
// permission.Type = "user";
// permission.EmailAddress = EmailAddress;
// permission.Role = UserRole;
// Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, fileId);
// createRequestPermission.TransferOwnership = true;
// createRequestPermission.Execute();
//}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
public static string CreateFolderWithPermission(string FolderName, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
//Create Folder in Google Drive
Google.Apis.Drive.v3.DriveService service = GetService_v3();
var fileMetadata = new Google.Apis.Drive.v3.Data.File()
{
Name = FolderName,
MimeType = "application/vnd.google-apps.folder",
Description = "File_" + System.DateTime.Now.Hour + ":" + System.DateTime.Now.Minute + ":" + System.DateTime.Now.Second
};
Google.Apis.Drive.v3.FilesResource.CreateRequest request = service.Files.Create(fileMetadata);
request.Fields = "id";
string Fileid = request.Execute().Id;
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
permission = service.Permissions.Create(permission, Fileid).Execute();
}
else if (UserRole == "owner")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, Fileid);
createRequestPermission.TransferOwnership = true;
createRequestPermission.Execute();
}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
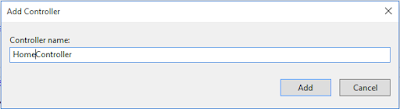
Copy & Paste this code into this controller:
using GoogleDriveRestAPI_v3.Models;
using System;
using System.Web;
using System.Web.Mvc;
using System.Linq;
using System.Collections.Generic;
using System.IO;
namespace GoogleDriveRestAPI_v3.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpPost]
public ActionResult UploadFile(HttpPostedFileBase file)
{
GoogleDriveFilesRepository.FileUpload(file);
return RedirectToAction("GetGoogleDriveFiles");
}
[HttpGet]
public string FileSharePermission(string fileId, string permissionValue, string PermissionType, string UserRole)
{
string Result = GoogleDriveFilesRepository.FileSharePermission(fileId, permissionValue, UserRole);
return Result;
}
[HttpGet]
public string CreateFolderWithPermission(string FolderName, string EmailAddress, string PermissionType, string UserRole)
{
string Result = GoogleDriveFilesRepository.CreateFolderWithPermission(FolderName, EmailAddress, UserRole);
return Result;
}
public JsonResult Render_GetGoogleDriveFilesView()
{
Dictionary<string, object> jsonResult = new Dictionary<string, object>();
var result = GoogleDriveFilesRepository.GetDriveFiles();
jsonResult.Add("Html", RenderRazorViewToString("~/Views/Home/GetGoogleDriveFiles.cshtml", result));
return Json(jsonResult, JsonRequestBehavior.AllowGet);
}
public string RenderRazorViewToString(string viewName, object model)
{
if (model != null)
{
ViewData.Model = model;
}
using (var sw = new StringWriter())
{
var viewResult = ViewEngines.Engines.FindPartialView(ControllerContext, viewName);
var viewContext = new ViewContext(ControllerContext, viewResult.View, ViewData, TempData, sw);
viewResult.View.Render(viewContext, sw);
viewResult.ViewEngine.ReleaseView(ControllerContext, viewResult.View);
return sw.GetStringBuilder().ToString();
}
}
}
}
Step 6 (View): We need to create one view, this view for showing the root directory contains Google drive.
View : Add a View (View Name: GetGoogleDriveFiles.cshtml) of GetGoogleDriveFiles action method.
Copy & Paste this code within this View:
@model IEnumerable<GoogleDriveRestAPI_v3.Models.GoogleDriveFiles>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<div id="Render_GetGoogleDriveFilesView">
<h2 class="imagetable">Google Drive API v3 - [Root Directory]</h2>
<center>
<div style="width:100%;">
<table class="imagetable">
<tr>
<td>
<h3>Create Folder with Share Permission</h3>
<p>
Email Address : <input type="text" id="EmailAddress" style="align-content:center" />
</p>
<p>
Folder Name : <input type="text" id="FolderName" style="align-content:center" />
Permission Type :<select id="UserRole">
<option>--Select--</option>
<option value="reader">reader</option>
<option value="writer">writer</option>
<option value="owner">owner</option>
</select>
<input type="button" id="ShareFolder" value="Create Folder" style="align-content:center" />
</p>
</td>
<td>
@using (Html.BeginForm("UploadFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<label>File Upload</label>
<input type="file" name="file" id="file" style="width:176px;" />
<input type="submit" value="Upload" />
}
</td>
</tr>
</table>
</div>
<br />
<table class="imagetable">
<tr id="header">
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
Type
</th>
<th>
Set Permission to Share
</th>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
if (item.Parents[0] == "0ANNR3nwrf6gUUk9PVA")
{
<tr>
@{
string FileSize = string.Empty;
string Type = string.Empty;
if (@item.Size != null)
{
long? KiloByte = @item.Size;
FileSize = KiloByte + " bytes";
}
else
{
FileSize = "0 bytes";
}
}
<td>
@item.Name
</td>
<td>
@{
if (FileSize == "0 bytes") { Type = "Folder"; }
else { Type = "File"; }
}
@Type
</td>
<td>
<input type="text" id="@item.Id" placeholder="email address" />
<select id='UserRole_@item.Id'>
<option>--Select--</option>
<option value="reader">reader</option>
<option value="writer">writer</option>
<option value="owner">owner</option>
</select>
<input type="button" class="ShareLink" data-key="@item.Id" value="Get Shareable Link" style="align-content:center" />
</td>
</tr>
}
}
}
else
{
<tr> <td colspan="6">No Records found</td> </tr>
}
</table>
</center>
</div>
<script type="text/javascript">
$(document).on('click', '#ShareFolder', function () {
debugger;
var EmailAddress = $("#EmailAddress").val();
var FolderName = $("#FolderName").val();
var UserRole = $("#UserRole").find("option:selected").attr("value");
$.ajax({
url: '/Home/CreateFolderWithPermission',
type: "GET",
contentType: "application/json",
data: { FolderName: FolderName, EmailAddress: EmailAddress, UserRole: UserRole},
success: function (result) {
if (result == 'Success') {
var ShareLink = 'Folder Name: [' + FolderName + '] Successfully Shared with [' + EmailAddress + ']';
ReloadView();
alert(ShareLink);
}
else {
alert(result);
}
},
error: function (jqXHR, textStatus, errorThrown) {
alert(textStatus);
}
});
});
$(document).on('click', '.ShareLink', function () {
debugger;
var FileId = $(this).attr("data-key");
var PermissionValue = $('#' + FileId).val();
var UserRole = $("#UserRole_" + FileId).find("option:selected").attr("value");
$.ajax({
url: '/Home/FileSharePermission',
type: "GET",
contentType: "application/json",
data: { fileId: FileId, permissionValue: PermissionValue, UserRole: UserRole },
success: function (result) {
var ShareLink = 'https://drive.google.com/open?id=' + FileId;
ReloadView();
alert(ShareLink);
},
error: function (jqXHR, textStatus, errorThrown) {
alert(textStatus);
}
});
});
function ReloadView() {
$("#Render_GetGoogleDriveFilesView").html("<center><img src='../../Images/ajax-loading.gif' alt='Loading...' /></center>");
$.ajax({
url: "/Home/Render_GetGoogleDriveFilesView",
type: "POST",
contentType: "application/json",
success: function (data) {
$("#Render_GetGoogleDriveFilesView").html(data.Html);
}
});
}
</script>
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
If you want to share a files or folder in google drive, you need to set the permission of file & folders at the time of uploading or after uploading a file in google drive.
Programmatic we can Insert, Update, Delete drive item permission to file/folder in google drive using Google Drive Rest API in v3.
In this video, I will discuss only Insert drive item permission to file/folder in google drive.
In my upcoming tutorials, I will discuss "how to Update, Delete drive item permission to file/folders"
Programmatically permission can effect in mainly four property of drive API v3 Permission class
1) User Type
2) User Role
3) EmailAddress
4) Domain
a) user - email address - for example everydaybecoding@gmail.com
b) group - group email address - for example GroupName@googlegroup.com
c) domain - domain name of G-Suit - for example thecompany.com
d) anyone -N/A - no permission is requird.
What is Google Group?
Google Groups is a group of people. such as project teams, departments & classmates. we can send an email to everyone in a group with one address, invite a group to an event, or share documents with a group.
2) User Role is classified as four type:
a) reader - only read the content, metadata of file & folder (like - name & description).
b) writer - all type of permission but not allow for delete file & folders.
c) owner - almost all type of permission but not allowed in Team Drives.
d) organizer - all type of Role is only valid for Google Team Drives.
For more Information about file ownership, please visit this link:
https://support.google.com/drive/answer/2494892?co=GENIE.Platform%3DDesktop&hl=en
What is Google Team Drive?
Google Team Drives are shared spaces where teams can easily store, search, and access their files anywhere, from any device.
Unlike files in My Drive, files in Team Drive belong to the team instead of an individual. Even if members leave, the files stay exactly where they are so your team can continue to share information and get work done.
For More Information visit this link:
https://gsuite.google.com/learning-center/products/drive/get-started-team-drive/
3) EmailAddress : Email address of User & Google groups. This property is present when permission type is user & groups.
4) Domain: Use any Google domain address, For Example, admin@company.com.
For More Information visit this link:
https://developers.google.com/drive/v3/web/about-permissions
A) Create a folder with Share Permission.
Please see this code:
public static string CreateFolderWithPermission(string FolderName, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
//Create Folder in Google Drive
Google.Apis.Drive.v3.DriveService service = GetService_v3();
var fileMetadata = new Google.Apis.Drive.v3.Data.File()
{
Name = FolderName,
MimeType = "application/vnd.google-apps.folder",
Description = "File_" + System.DateTime.Now.Hour + ":" + System.DateTime.Now.Minute + ":" + System.DateTime.Now.Second
};
Google.Apis.Drive.v3.FilesResource.CreateRequest request = service.Files.Create(fileMetadata);
request.Fields = "id";
string Fileid = request.Execute().Id;
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
permission = service.Permissions.Create(permission, Fileid).Execute();
}
else if (UserRole == "owner")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, Fileid);
createRequestPermission.TransferOwnership = true;
createRequestPermission.Execute();
}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
As a 'reader','writer' you can share any file that you want, but in case of 'owner', you can only transfer ownership of Google files or Google items.
These are Google Docs, Google Sheets, Google Slides, Google Forms, Google Drawing, Google My Maps & Folders.
public static string FileSharePermission(string fileId, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user"; //'user', 'group', 'domain', 'anyone' - all are small letter keyword
permission.EmailAddress = EmailAddress; // 'reader', 'writer', 'owner', 'organizer' - all are small letter keyword
permission.Role = UserRole; // use any Google account: emails or groups
permission = service.Permissions.Create(permission, fileId).Execute();
}
//else if (UserRole == "owner")
//{
// permission.Type = "user";
// permission.EmailAddress = EmailAddress;
// permission.Role = UserRole;
// Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, fileId);
// createRequestPermission.TransferOwnership = true;
// createRequestPermission.Execute();
//}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
Let's do it using Visual Studio MVC Application:
First, Goto the Visual Studio 2013 or Higher Version.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following command.
PM> Install-Package Google.Apis.Drive.v3
Here I am using Google Drive API v3,
First, we need to create one model class under the Model folder of project solution.
Model Class Name: GoogleDriveFiles.cs
Please Copy & Paste this code into this class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public IList<string> Parents { get; set; }
}
}
Step 4 (Repository):
After that, create another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Please Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFilesRepository
{
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//Create Drive API service.
Google.Apis.Drive.v3.DriveService service = new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(createdTime, id, name, size, version, trashed, parents, webViewLink)";
// Request of List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents,
};
FileList.Add(File);
}
}
return FileList;
}
public static void FileUpload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
string path = Path.Combine(HttpContext.Current.Server.MapPath("~/GoogleDriveFiles"),
Path.GetFileName(file.FileName));
file.SaveAs(path);
Google.Apis.Drive.v3.Data.File FileMetaData = new Google.Apis.Drive.v3.Data.File();
FileMetaData.Name = Path.GetFileName(file.FileName);
FileMetaData.MimeType = MimeMapping.GetMimeMapping(path);
Google.Apis.Drive.v3.FilesResource.CreateMediaUpload request;
using (var stream = new System.IO.FileStream(path, System.IO.FileMode.Open))
{
request = service.Files.Create(FileMetaData, stream, FileMetaData.MimeType);
request.Fields = "id";
request.Upload();
}
}
}
public static string FileSharePermission(string fileId, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user"; //'user', 'group', 'domain', 'anyone' - all are small letter keyword
permission.EmailAddress = EmailAddress; // 'reader', 'writer', 'owner', 'organizer' - all are small letter keyword
permission.Role = UserRole; // use any Google account: emails or groups
permission = service.Permissions.Create(permission, fileId).Execute();
}
//else if (UserRole == "owner")
//{
// permission.Type = "user";
// permission.EmailAddress = EmailAddress;
// permission.Role = UserRole;
// Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, fileId);
// createRequestPermission.TransferOwnership = true;
// createRequestPermission.Execute();
//}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
public static string CreateFolderWithPermission(string FolderName, string EmailAddress, string UserRole)
{
string message = string.Empty;
try
{
//Create Folder in Google Drive
Google.Apis.Drive.v3.DriveService service = GetService_v3();
var fileMetadata = new Google.Apis.Drive.v3.Data.File()
{
Name = FolderName,
MimeType = "application/vnd.google-apps.folder",
Description = "File_" + System.DateTime.Now.Hour + ":" + System.DateTime.Now.Minute + ":" + System.DateTime.Now.Second
};
Google.Apis.Drive.v3.FilesResource.CreateRequest request = service.Files.Create(fileMetadata);
request.Fields = "id";
string Fileid = request.Execute().Id;
Google.Apis.Drive.v3.Data.Permission permission = new Google.Apis.Drive.v3.Data.Permission();
if (UserRole == "writer" || UserRole == "reader")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
permission = service.Permissions.Create(permission, Fileid).Execute();
}
else if (UserRole == "owner")
{
permission.Type = "user";
permission.EmailAddress = EmailAddress;
permission.Role = UserRole;
Google.Apis.Drive.v3.PermissionsResource.CreateRequest createRequestPermission = service.Permissions.Create(permission, Fileid);
createRequestPermission.TransferOwnership = true;
createRequestPermission.Execute();
}
if (permission != null)
{
message = "Success";
}
}
catch (Exception e)
{
message = "An error occurred: " + e.Message;
}
return message;
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
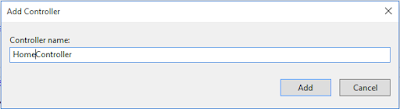
Copy & Paste this code into this controller:
using GoogleDriveRestAPI_v3.Models;
using System;
using System.Web;
using System.Web.Mvc;
using System.Linq;
using System.Collections.Generic;
using System.IO;
namespace GoogleDriveRestAPI_v3.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpPost]
public ActionResult UploadFile(HttpPostedFileBase file)
{
GoogleDriveFilesRepository.FileUpload(file);
return RedirectToAction("GetGoogleDriveFiles");
}
[HttpGet]
public string FileSharePermission(string fileId, string permissionValue, string PermissionType, string UserRole)
{
string Result = GoogleDriveFilesRepository.FileSharePermission(fileId, permissionValue, UserRole);
return Result;
}
[HttpGet]
public string CreateFolderWithPermission(string FolderName, string EmailAddress, string PermissionType, string UserRole)
{
string Result = GoogleDriveFilesRepository.CreateFolderWithPermission(FolderName, EmailAddress, UserRole);
return Result;
}
public JsonResult Render_GetGoogleDriveFilesView()
{
Dictionary<string, object> jsonResult = new Dictionary<string, object>();
var result = GoogleDriveFilesRepository.GetDriveFiles();
jsonResult.Add("Html", RenderRazorViewToString("~/Views/Home/GetGoogleDriveFiles.cshtml", result));
return Json(jsonResult, JsonRequestBehavior.AllowGet);
}
public string RenderRazorViewToString(string viewName, object model)
{
if (model != null)
{
ViewData.Model = model;
}
using (var sw = new StringWriter())
{
var viewResult = ViewEngines.Engines.FindPartialView(ControllerContext, viewName);
var viewContext = new ViewContext(ControllerContext, viewResult.View, ViewData, TempData, sw);
viewResult.View.Render(viewContext, sw);
viewResult.ViewEngine.ReleaseView(ControllerContext, viewResult.View);
return sw.GetStringBuilder().ToString();
}
}
}
}
Step 6 (View): We need to create one view, this view for showing the root directory contains Google drive.
View : Add a View (View Name: GetGoogleDriveFiles.cshtml) of GetGoogleDriveFiles action method.
Copy & Paste this code within this View:
@model IEnumerable<GoogleDriveRestAPI_v3.Models.GoogleDriveFiles>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<div id="Render_GetGoogleDriveFilesView">
<h2 class="imagetable">Google Drive API v3 - [Root Directory]</h2>
<center>
<div style="width:100%;">
<table class="imagetable">
<tr>
<td>
<h3>Create Folder with Share Permission</h3>
<p>
Email Address : <input type="text" id="EmailAddress" style="align-content:center" />
</p>
<p>
Folder Name : <input type="text" id="FolderName" style="align-content:center" />
Permission Type :<select id="UserRole">
<option>--Select--</option>
<option value="reader">reader</option>
<option value="writer">writer</option>
<option value="owner">owner</option>
</select>
<input type="button" id="ShareFolder" value="Create Folder" style="align-content:center" />
</p>
</td>
<td>
@using (Html.BeginForm("UploadFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<label>File Upload</label>
<input type="file" name="file" id="file" style="width:176px;" />
<input type="submit" value="Upload" />
}
</td>
</tr>
</table>
</div>
<br />
<table class="imagetable">
<tr id="header">
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
Type
</th>
<th>
Set Permission to Share
</th>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
if (item.Parents[0] == "0ANNR3nwrf6gUUk9PVA")
{
<tr>
@{
string FileSize = string.Empty;
string Type = string.Empty;
if (@item.Size != null)
{
long? KiloByte = @item.Size;
FileSize = KiloByte + " bytes";
}
else
{
FileSize = "0 bytes";
}
}
<td>
@item.Name
</td>
<td>
@{
if (FileSize == "0 bytes") { Type = "Folder"; }
else { Type = "File"; }
}
@Type
</td>
<td>
<input type="text" id="@item.Id" placeholder="email address" />
<select id='UserRole_@item.Id'>
<option>--Select--</option>
<option value="reader">reader</option>
<option value="writer">writer</option>
<option value="owner">owner</option>
</select>
<input type="button" class="ShareLink" data-key="@item.Id" value="Get Shareable Link" style="align-content:center" />
</td>
</tr>
}
}
}
else
{
<tr> <td colspan="6">No Records found</td> </tr>
}
</table>
</center>
</div>
<script type="text/javascript">
$(document).on('click', '#ShareFolder', function () {
debugger;
var EmailAddress = $("#EmailAddress").val();
var FolderName = $("#FolderName").val();
var UserRole = $("#UserRole").find("option:selected").attr("value");
$.ajax({
url: '/Home/CreateFolderWithPermission',
type: "GET",
contentType: "application/json",
data: { FolderName: FolderName, EmailAddress: EmailAddress, UserRole: UserRole},
success: function (result) {
if (result == 'Success') {
var ShareLink = 'Folder Name: [' + FolderName + '] Successfully Shared with [' + EmailAddress + ']';
ReloadView();
alert(ShareLink);
}
else {
alert(result);
}
},
error: function (jqXHR, textStatus, errorThrown) {
alert(textStatus);
}
});
});
$(document).on('click', '.ShareLink', function () {
debugger;
var FileId = $(this).attr("data-key");
var PermissionValue = $('#' + FileId).val();
var UserRole = $("#UserRole_" + FileId).find("option:selected").attr("value");
$.ajax({
url: '/Home/FileSharePermission',
type: "GET",
contentType: "application/json",
data: { fileId: FileId, permissionValue: PermissionValue, UserRole: UserRole },
success: function (result) {
var ShareLink = 'https://drive.google.com/open?id=' + FileId;
ReloadView();
alert(ShareLink);
},
error: function (jqXHR, textStatus, errorThrown) {
alert(textStatus);
}
});
});
function ReloadView() {
$("#Render_GetGoogleDriveFilesView").html("<center><img src='../../Images/ajax-loading.gif' alt='Loading...' /></center>");
$.ajax({
url: "/Home/Render_GetGoogleDriveFilesView",
type: "POST",
contentType: "application/json",
success: function (data) {
$("#Render_GetGoogleDriveFilesView").html(data.Html);
}
});
}
</script>
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
YouTube : | https://goo.gl/rt4tHH |
Facebook : | https://goo.gl/hgpQsh |
Twitter : | https://goo.gl/nUwGnf |
Thank you. Great post..
ReplyDelete