Cloud Storage Access through API - ASP.NET MVC 5 - C# (Part-2)
Google Drive API v3 - a) File Uploading,
b) Viewing
c) Downloading &
d) Deleting
Google Drive API v3 - a) File Uploading,
b) Viewing
c) Downloading &
d) Deleting
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
This Video: Click here
Source Code: Click to download [10.4 MB]
In Part-1 of this video series we have seen "How to Enable Google Drive API v3 for your application". After enabling API, a JSON(JavaScript Object Notation) file (client_secret.json) provided by Google. The contains of this files are clients information.
These are some keys & its value a) client id,
b) client secret id,
c) project_id
d) auth_uri,
e) token_uri
f) redirect_uris
The key & value information are extremely useful for every time your application communication with Google Drive APIs.
A) Attach client secret Json file:
Please copy this downloaded client_secret.json file & paste into any path of your hard disk:
In my case: D:\
Now, Go to the Visual Studio 2013 or Higher version:
Step 1: First Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI_v3
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following command.
Step 3 (Model):
Create a model class (Name: GoogleDriveFiles.cs) under the Model folder of project solution. Copy & Paste this code into this class.
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public long? Version { get; set; }
public DateTime? CreatedTime { get; set; }
}
}
B) Drive Access Permission Scope of Application:
Here, we need to get permission to our application.
DriveService.Scope.Drive: View and manage the files in your Google Drive.
DriveService.Scope.DriveAppdata: View and manage its own configuration data in your Google Drive.
DriveService.Scope.DriveFile: View and manage google drive files and folders that your are open and created with this app.
DriveService.Scope.DriveMetadata: View and manage metadata of files in your Google Drive
DriveService.Scope.Drive.MetadataReadonly: View metadata of files in your Google Drive.
DriveService.Scope.Drive.PhotosReadonly: views the photos, videos, albums in your Google Photos.
DriveService.Scope.Drive.ReadOnly: View the files in your Google Drive.
DriveService.Scope.DriveScripts: Modify your Google apps Scripts script behavior.
Step 4 (Repository):
After that, create an another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Download;
using Google.Apis.Drive.v3;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFilesRepository
{
//defined scope.
public static string[] Scopes = { DriveService.Scope.Drive };
//create Drive API service.
public static DriveService GetService()
{
//get Credentials from client_secret.json file
UserCredential credential;
using (var stream = new FileStream( @"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//create Drive API service.
DriveService service = new DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
//get all files from Google Drive.
public static List<GoogleDriveFiles> GetDriveFiles()
{
DriveService service = GetService();
// define parameters of request.
FilesResource.ListRequest FileListRequest = service.Files.List();
//listRequest.PageSize = 10;
//listRequest.PageToken = 10;
FileListRequest.Fields = "nextPageToken, files(id, name, size, version, createdTime)";
//get file list.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles {
Id = file.Id,
Name = file.Name,
Size = file.Size,
Version = file.Version,
CreatedTime = file.CreatedTime
};
FileList.Add(File);
}
}
return FileList;
}
//file Upload to the Google Drive.
public static void FileUpload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
DriveService service = GetService();
string path = Path.Combine(HttpContext.Current.Server.MapPath("~/GoogleDriveFiles"),
Path.GetFileName(file.FileName));
file.SaveAs(path);
var FileMetaData = new Google.Apis.Drive.v3.Data.File();
FileMetaData.Name = Path.GetFileName(file.FileName);
FileMetaData.MimeType = MimeMapping.GetMimeMapping(path);
FilesResource.CreateMediaUpload request;
using (var stream = new System.IO.FileStream(path, System.IO.FileMode.Open))
{
request = service.Files.Create(FileMetaData, stream, FileMetaData.MimeType);
request.Fields = "id";
request.Upload();
}
}
}
//Download file from Google Drive by fileId.
public static string DownloadGoogleFile(string fileId)
{
DriveService service = GetService();
string FolderPath = System.Web.HttpContext.Current.Server.MapPath("/GoogleDriveFiles/");
FilesResource.GetRequest request = service.Files.Get(fileId);
string FileName = request.Execute().Name;
string FilePath = System.IO.Path.Combine(FolderPath, FileName);
MemoryStream stream1 = new MemoryStream();
// Add a handler which will be notified on progress changes.
// It will notify on each chunk download and when the
// download is completed or failed.
request.MediaDownloader.ProgressChanged += (Google.Apis.Download.IDownloadProgress progress) =>
{
switch (progress.Status)
{
case DownloadStatus.Downloading:
{
Console.WriteLine(progress.BytesDownloaded);
break;
}
case DownloadStatus.Completed:
{
Console.WriteLine("Download complete.");
SaveStream(stream1, FilePath);
break;
}
case DownloadStatus.Failed:
{
Console.WriteLine("Download failed.");
break;
}
}
};
request.Download(stream1);
return FilePath;
}
// file save to server path
private static void SaveStream(MemoryStream stream, string FilePath)
{
using (System.IO.FileStream file = new FileStream(FilePath, FileMode.Create, FileAccess.ReadWrite))
{
stream.WriteTo(file);
}
}
//Delete file from the Google drive
public static void DeleteFile(GoogleDriveFiles files)
{
DriveService service = GetService();
try
{
// Initial validation.
if (service == null)
throw new ArgumentNullException("service");
if (files == null)
throw new ArgumentNullException(files.Id);
// Make the request.
service.Files.Delete(files.Id).Execute();
}
catch (Exception ex)
{
throw new Exception("Request Files.Delete failed.", ex);
}
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
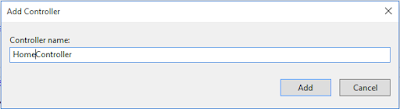
Copy & Paste this code this code within this controller:
using GoogleDriveRestAPI_v3.Models;
using System.IO;
using System.Web;
using System.Web.Mvc;
namespace GoogleDriveRestAPI_v3.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpPost]
public ActionResult DeleteFile(GoogleDriveFiles file)
{
GoogleDriveFilesRepository.DeleteFile(file);
return RedirectToAction("GetGoogleDriveFiles");
}
[HttpPost]
public ActionResult UploadFile(HttpPostedFileBase file)
{
GoogleDriveFilesRepository.FileUpload(file);
return RedirectToAction("GetGoogleDriveFiles");
}
public void DownloadFile(string id)
{
string FilePath = GoogleDriveFilesRepository.DownloadGoogleFile(id);
Response.ContentType = "application/zip";
Response.AddHeader("Content-Disposition", "attachment; filename=" + Path.GetFileName(FilePath));
Response.WriteFile(System.Web.HttpContext.Current.Server.MapPath("~/GoogleDriveFiles/" + Path.GetFileName(FilePath)));
Response.End();
Response.Flush();
}
}
}
Step 6 (View): Add a View (View Name: GetGoogleDriveFiles.cshtml) of
GetGoogleDriveFiles action method.
@model IEnumerable<GoogleDriveRestAPI_v3.Models.GoogleDriveFiles>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<h2>Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]</h2>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<style type="text/css">
#header {
width: 100%;
background-color: #CCCCCC;
text-align: center;
}
#layouttable {
border: 0px;
width: 100%;
font-family: 'Segoe UI';
}
#layouttable td.col1 {
width: 20%;
vertical-align: top;
}
#layouttable td.col2 {
width: 60%;
vertical-align: top;
background-color: #E8E8E8;
}
#layouttable td.col3 {
width: 20%;
vertical-align: top;
}
</style>
<center>
<div style="width:80%; text-align:left;">
@using (Html.BeginForm("UploadFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<p>
<label for="file">Upload file:</label>
<input type="file" name="file" id="file" />
<input type="submit" value="Upload"/>
</p>
}
</div>
<table class="table" border="1">
<tr id="header">
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Size)
</th>
<th>
@Html.DisplayNameFor(model => model.Version)
</th>
<th>
@Html.DisplayNameFor(model => model.CreatedTime)
</th>
<th>
Download
</th>
<th>
Delete
</th>
</tr>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
<tr id="layouttable">
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@{
long? KiloByte = @item.Size/1024;
string NewSize = KiloByte + " KB";
}
@NewSize
</td>
<td>
@Html.DisplayFor(modelItem => item.Version)
</td>
<td>
@string.Format("{0: MM/dd/yyyy}", Convert.ToDateTime(Html.DisplayFor(modelItem => item.CreatedTime).ToString()))
</td>
<td>
<input type="button" class="DownloadFile" value="Download" data-key=@item.Id style="align-content:center" />
</td>
<td>
@using (Html.BeginForm("DeleteFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="hidden" name=Id value="@item.Id">
<input type="submit" class="DeleteFile" value="Delete" style="align-content:center" />
}
</td>
</tr>
}
}
else
{
<td colspan="6">No files found</td>
}
</table>
</center>
<script>
$(document).on('click', '.DownloadFile', function () {
debugger;
var fileId = $(this).attr("data-key");
window.location.href = '/Home/DownloadFile/' + fileId;
});
</script>
Step 7 (View): Change the default action method name 'Index' to 'GetGoogleDriveFiles'.
using System.Web.Mvc;
using System.Web.Routing;
namespace GoogleDriveRestAPI_v3
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "GetGoogleDriveFiles", id = UrlParameter.Optional }
);
}
}
}
These are some keys & its value a) client id,
b) client secret id,
c) project_id
d) auth_uri,
e) token_uri
f) redirect_uris
The key & value information are extremely useful for every time your application communication with Google Drive APIs.
A) Attach client secret Json file:
Please copy this downloaded client_secret.json file & paste into any path of your hard disk:
In my case: D:\
Now, Go to the Visual Studio 2013 or Higher version:
Step 1: First Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI_v3
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following command.
Create a model class (Name: GoogleDriveFiles.cs) under the Model folder of project solution. Copy & Paste this code into this class.
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public long? Version { get; set; }
public DateTime? CreatedTime { get; set; }
}
}
B) Drive Access Permission Scope of Application:
Here, we need to get permission to our application.
DriveService.Scope.Drive: View and manage the files in your Google Drive.
DriveService.Scope.DriveAppdata: View and manage its own configuration data in your Google Drive.
DriveService.Scope.DriveFile: View and manage google drive files and folders that your are open and created with this app.
DriveService.Scope.DriveMetadata: View and manage metadata of files in your Google Drive
DriveService.Scope.Drive.MetadataReadonly: View metadata of files in your Google Drive.
DriveService.Scope.Drive.PhotosReadonly: views the photos, videos, albums in your Google Photos.
DriveService.Scope.Drive.ReadOnly: View the files in your Google Drive.
DriveService.Scope.DriveScripts: Modify your Google apps Scripts script behavior.
Step 4 (Repository):
After that, create an another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Download;
using Google.Apis.Drive.v3;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFilesRepository
{
//defined scope.
public static string[] Scopes = { DriveService.Scope.Drive };
//create Drive API service.
public static DriveService GetService()
{
//get Credentials from client_secret.json file
UserCredential credential;
using (var stream = new FileStream( @"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//create Drive API service.
DriveService service = new DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
//get all files from Google Drive.
public static List<GoogleDriveFiles> GetDriveFiles()
{
DriveService service = GetService();
// define parameters of request.
FilesResource.ListRequest FileListRequest = service.Files.List();
//listRequest.PageSize = 10;
//listRequest.PageToken = 10;
FileListRequest.Fields = "nextPageToken, files(id, name, size, version, createdTime)";
//get file list.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles {
Id = file.Id,
Name = file.Name,
Size = file.Size,
Version = file.Version,
CreatedTime = file.CreatedTime
};
FileList.Add(File);
}
}
return FileList;
}
//file Upload to the Google Drive.
public static void FileUpload(HttpPostedFileBase file)
{
if (file != null && file.ContentLength > 0)
{
DriveService service = GetService();
string path = Path.Combine(HttpContext.Current.Server.MapPath("~/GoogleDriveFiles"),
Path.GetFileName(file.FileName));
file.SaveAs(path);
var FileMetaData = new Google.Apis.Drive.v3.Data.File();
FileMetaData.Name = Path.GetFileName(file.FileName);
FileMetaData.MimeType = MimeMapping.GetMimeMapping(path);
FilesResource.CreateMediaUpload request;
using (var stream = new System.IO.FileStream(path, System.IO.FileMode.Open))
{
request = service.Files.Create(FileMetaData, stream, FileMetaData.MimeType);
request.Fields = "id";
request.Upload();
}
}
}
//Download file from Google Drive by fileId.
public static string DownloadGoogleFile(string fileId)
{
DriveService service = GetService();
string FolderPath = System.Web.HttpContext.Current.Server.MapPath("/GoogleDriveFiles/");
FilesResource.GetRequest request = service.Files.Get(fileId);
string FileName = request.Execute().Name;
string FilePath = System.IO.Path.Combine(FolderPath, FileName);
MemoryStream stream1 = new MemoryStream();
// Add a handler which will be notified on progress changes.
// It will notify on each chunk download and when the
// download is completed or failed.
request.MediaDownloader.ProgressChanged += (Google.Apis.Download.IDownloadProgress progress) =>
{
switch (progress.Status)
{
case DownloadStatus.Downloading:
{
Console.WriteLine(progress.BytesDownloaded);
break;
}
case DownloadStatus.Completed:
{
Console.WriteLine("Download complete.");
SaveStream(stream1, FilePath);
break;
}
case DownloadStatus.Failed:
{
Console.WriteLine("Download failed.");
break;
}
}
};
request.Download(stream1);
return FilePath;
}
// file save to server path
private static void SaveStream(MemoryStream stream, string FilePath)
{
using (System.IO.FileStream file = new FileStream(FilePath, FileMode.Create, FileAccess.ReadWrite))
{
stream.WriteTo(file);
}
}
//Delete file from the Google drive
public static void DeleteFile(GoogleDriveFiles files)
{
DriveService service = GetService();
try
{
// Initial validation.
if (service == null)
throw new ArgumentNullException("service");
if (files == null)
throw new ArgumentNullException(files.Id);
// Make the request.
service.Files.Delete(files.Id).Execute();
}
catch (Exception ex)
{
throw new Exception("Request Files.Delete failed.", ex);
}
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
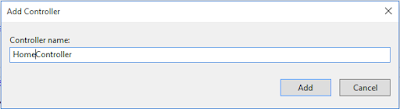
Copy & Paste this code this code within this controller:
using GoogleDriveRestAPI_v3.Models;
using System.IO;
using System.Web;
using System.Web.Mvc;
namespace GoogleDriveRestAPI_v3.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpPost]
public ActionResult DeleteFile(GoogleDriveFiles file)
{
GoogleDriveFilesRepository.DeleteFile(file);
return RedirectToAction("GetGoogleDriveFiles");
}
[HttpPost]
public ActionResult UploadFile(HttpPostedFileBase file)
{
GoogleDriveFilesRepository.FileUpload(file);
return RedirectToAction("GetGoogleDriveFiles");
}
public void DownloadFile(string id)
{
string FilePath = GoogleDriveFilesRepository.DownloadGoogleFile(id);
Response.ContentType = "application/zip";
Response.AddHeader("Content-Disposition", "attachment; filename=" + Path.GetFileName(FilePath));
Response.WriteFile(System.Web.HttpContext.Current.Server.MapPath("~/GoogleDriveFiles/" + Path.GetFileName(FilePath)));
Response.End();
Response.Flush();
}
}
}
Step 6 (View): Add a View (View Name: GetGoogleDriveFiles.cshtml) of
GetGoogleDriveFiles action method.
@model IEnumerable<GoogleDriveRestAPI_v3.Models.GoogleDriveFiles>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<h2>Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]</h2>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<style type="text/css">
#header {
width: 100%;
background-color: #CCCCCC;
text-align: center;
}
#layouttable {
border: 0px;
width: 100%;
font-family: 'Segoe UI';
}
#layouttable td.col1 {
width: 20%;
vertical-align: top;
}
#layouttable td.col2 {
width: 60%;
vertical-align: top;
background-color: #E8E8E8;
}
#layouttable td.col3 {
width: 20%;
vertical-align: top;
}
</style>
<center>
<div style="width:80%; text-align:left;">
@using (Html.BeginForm("UploadFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<p>
<label for="file">Upload file:</label>
<input type="file" name="file" id="file" />
<input type="submit" value="Upload"/>
</p>
}
</div>
<table class="table" border="1">
<tr id="header">
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Size)
</th>
<th>
@Html.DisplayNameFor(model => model.Version)
</th>
<th>
@Html.DisplayNameFor(model => model.CreatedTime)
</th>
<th>
Download
</th>
<th>
Delete
</th>
</tr>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
<tr id="layouttable">
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@{
long? KiloByte = @item.Size/1024;
string NewSize = KiloByte + " KB";
}
@NewSize
</td>
<td>
@Html.DisplayFor(modelItem => item.Version)
</td>
<td>
@string.Format("{0: MM/dd/yyyy}", Convert.ToDateTime(Html.DisplayFor(modelItem => item.CreatedTime).ToString()))
</td>
<td>
<input type="button" class="DownloadFile" value="Download" data-key=@item.Id style="align-content:center" />
</td>
<td>
@using (Html.BeginForm("DeleteFile", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<input type="hidden" name=Id value="@item.Id">
<input type="submit" class="DeleteFile" value="Delete" style="align-content:center" />
}
</td>
</tr>
}
}
else
{
<td colspan="6">No files found</td>
}
</table>
</center>
<script>
$(document).on('click', '.DownloadFile', function () {
debugger;
var fileId = $(this).attr("data-key");
window.location.href = '/Home/DownloadFile/' + fileId;
});
</script>
Step 7 (View): Change the default action method name 'Index' to 'GetGoogleDriveFiles'.
using System.Web.Mvc;
using System.Web.Routing;
namespace GoogleDriveRestAPI_v3
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action = "GetGoogleDriveFiles", id = UrlParameter.Optional }
);
}
}
}
Output :
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
YouTube : | https://goo.gl/rt4tHH |
Facebook : | https://goo.gl/hgpQsh |
Twitter : | https://goo.gl/nUwGnf |
Please copy this downloaded client_secret.json file & paste into any path of your hard disk.
ReplyDeletei don't understand properly.where is client_secret.json?
ok mow i am understand.
ReplyDeleteThank you for the instruction, i did it, but after uploading i want to share the file for everyone. Can you help me?
ReplyDeleteThank you for your support
Thanks for your comment.
ReplyDeleteYou need to set permission when a file is first inserted/uploaded to Google drive.
In C# we can programmatic grant people permissions to files or folders on Google drive using the Google Drive API.
Programmatic you can set permission by
1) user Email or Domain Name
2) User Role
3) User Type
I will discuss this topics in my next video(Google Drive API #4). Thanks again...
Hi sir
Deletethanks for this wonderful article it work like a charm but sir i need to access all the folder and file from the google drive ad as per the code it access root director only.
can u suggest something thanks.
Hi i want to Known where is the file client_secret.json???(Please copy this downloaded client_secret.json file & paste into any path of your hard disk:
ReplyDeleteIn my case: D:\ )
Thank you for your comment.
Delete"client_secret.json" is provided by google. You need to download your "client_secret.json" file from google API console [for your google account].
Please follow this video link : https://youtu.be/xtqpWG5KDXY
I got this to work on localhost, but when deployed to production, nothing happens. There is not even a request going through, fiddler doesn't show any network activity, nor does the browser developer tool. I added my production website to the domain verification in google. What else am I missing? Please advise.
ReplyDeleteI am facing the same issue
DeleteI am facing the same issue
DeleteThanks for sharing the article. I am using gmail authentication for login purpose. By using this code I am having two main problems :
ReplyDelete1. User is loged in with authenticated gmail, While uploading it asks user to log in again.
2. Since access token is created so after sign out also it is uploading file in the same user's drive for which token is physically present.
Please share your email so that I can share my code.
Id did all but i'm always geting : "DownloadStatus.Failed" but i can the file name via "string FileName = m__request.Execute().Name;" instruction !!!!
ReplyDeleteHello and thanks for the guide. But is that possible to upload the file directly to google drive without saving first to server in GoogleDriveFiles folder?
ReplyDeleteHello and thanks for the guide. Could you please tell me is that possible to upload directly to google drive without saving first GoogleDriveFiles folder first?
ReplyDeleteHello Rajdip.. I really appreciate your great work. I've been learning google drive API with your tutorials. May I know if there are any big differences between google drive .net API and REST API because I'm told to use google drive .net API to do my assigned task. Thanks in advance. :)
ReplyDeleteHello Rajdip.. I really appreciate your great work. I've been learning google drive API with your tutorials. May I know if there are any big differences between google drive .net API and REST API because I'm told to use google drive .net API to do my assigned task. Thanks in advance. :)
ReplyDeleteSir, i am getting below error. Please help.
ReplyDeleteGoogle.Apis.Requests.RequestError Invalid Value [400]
Errors [ Message[Invalid Value] Location[q - parameter] Reason[invalid] Domain[global]
Sir, I am getting below error. Please help
ReplyDeleteGoogle.Apis.Requests.RequestError Invalid Value [400]
Errors [ Message[Invalid Value] Location[q - parameter] Reason[invalid] Domain[global]
Hello I am tying to implement your coding above , everything worked but while downloading i get error for those files like spreadsheet and docs that i created online google drive. Any solution.
ReplyDeleteI am not able to download spreadsheet file that i created online google drive but text file is downloading any eason for that??
ReplyDelete
ReplyDeleteHi, I am not able to create DriveServiceCredentials.json folder on Server.It is giving error as "One or more errors occurred. , at System.Threading.Tasks.Task`1.GetResultCore(Boolean waitCompletionNotification)" and does not allow to create tokenresponse file in it.But it is working on local.
Hi,
ReplyDeleteI have written a code to Upload and Download files using from Google Drive.
It is working very fine in Local, but when I deploy it on Server it is giving error in Download part where we use “GoogleWebAuthorizationBroker.AuthorizeAsync" method to get Service credentials. It gives following error
“Server Error in '/' Application.
________________________________________
Access is denied
Description: An unhandled exception occurred during the execution of the current web request. Please review the stack trace for more information about the error and where it originated in the code.
Exception Details: System.ComponentModel.Win32Exception: Access is denied
Source Error:
An unhandled exception was generated during the execution of the current web request. Information regarding the origin and location of the exception can be identified using the exception stack trace below.
Stack Trace:
[Win32Exception (0x80004005): Access is denied]
Microsoft.Runtime.CompilerServices.TaskAwaiter.ThrowForNonSuccess(Task task) +185
Microsoft.Runtime.CompilerServices.TaskAwaiter.HandleNonSuccess(Task task) +114
Google.Apis.Auth.OAuth2.d__1.MoveNext() +483
[AggregateException: One or more errors occurred.]
System.Threading.Tasks.Task`1.GetResultCore(Boolean waitCompletionNotification) +5836036
GoogleDriveRestAPI_v3.Models.GoogleDriveFilesRepository.GetService() +467
GoogleDriveRestAPI_v3.Models.GoogleDriveFilesRepository.DownloadGoogleFile(String fileId) +35
GoogleDriveRestAPI_v3.Controllers.HomeController.DownloadFile(String id) +356
System.Web.Mvc.<>c__DisplayClass1.b__0(ControllerBase controller, Object[] parameters) +15
System.Web.Mvc.ReflectedActionDescriptor.Execute(ControllerContext controllerContext, IDictionary`2 parameters) +242
System.Web.Mvc.ControllerActionInvoker.InvokeActionMethod(ControllerContext controllerContext, ActionDescriptor actionDescriptor, IDictionary`2 parameters) +39
System.Web.Mvc.Async.AsyncControllerActionInvoker.b__39(IAsyncResult asyncResult, ActionInvocation innerInvokeState) +12
System.Web.Mvc.Async.WrappedAsyncResult`2.CallEndDelegate(IAsyncResult asyncResult) +139
System.Web.Mvc.Async.AsyncInvocationWithFilters.b__3d() +112
System.Web.Mvc.Async.<>c__DisplayClass46.b__3f() +452
System.Web.Mvc.Async.<>c__DisplayClass33.b__32(IAsyncResult asyncResult) +15
System.Web.Mvc.Async.<>c__DisplayClass2b.b__1c() +37
System.Web.Mvc.Async.<>c__DisplayClass21.b__1e(IAsyncResult asyncResult) +241
System.Web.Mvc.Controller.b__1d(IAsyncResult asyncResult, ExecuteCoreState innerState) +29
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +111
System.Web.Mvc.Controller.EndExecuteCore(IAsyncResult asyncResult) +53
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +19
System.Web.Mvc.MvcHandler.b__5(IAsyncResult asyncResult, ProcessRequestState innerState) +51
System.Web.Mvc.Async.WrappedAsyncVoid`1.CallEndDelegate(IAsyncResult asyncResult) +111
System.Web.CallHandlerExecutionStep.System.Web.HttpApplication.IExecutionStep.Execute() +606
System.Web.HttpApplication.ExecuteStep(IExecutionStep step, Boolean& completedSynchronously) +288
________________________________________
Version Information: Microsoft .NET Framework Version:4.0.30319; ASP.NET Version:4.0.30319.33440”
Due to this error TokenResponse file is not getting created using following code
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,
Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)
).Result;
Please suggest solution so that this code works on Server after deploying in IIS.
Hi,
DeleteKalyani Shelar
Have you fix that ?
what was the issue ?
Thanks
Hi
DeleteI have the same problem, are you solved ?
thanks
Not Just copy, use your mind.
DeleteHi
ReplyDeletemy goodle drive api doest not work on port
could you please help me
Please help me on displaying google login page before view google sheet.
ReplyDeleteThanks.
How to do client_secret.json directory in product server?
ReplyDeletehi
ReplyDeleteStep 7 not clear, share the details, it will be more clear
this code is working fine for the local host but unable to right the Google.Apis.Auth.OAuth2.Responses.TokenResponse-user file to the server when I deploy the code
ReplyDeletethis code is working fine in localhost but when I deploy code to the server Google.Apis.Auth.OAuth2.Responses.TokenResponse-user file not creating
ReplyDeleteHi Rajdip,
ReplyDeleteI have been following your tutorial for quite some time and It worked like a charm in my project but I am having some issues.
It works fine on my local machine when I try to download some specific file, as it opens a consent screen and after authentication it never asks you to authenticate again.
I have deployed the application on windows server 2012 R2 hoping that It would work fine there but It does not. There is no consent screen as It does not open any screen, or sign of error when it goes to authenticate user.
It seems stuck somewhere and just keep going back and forth and there is no way I can know whats going on in the background process.
I have searched the web and people are talking about cancellation token or something related to that.
If you have any knowledge about this problem or any way to get out of this, please let me know.
Best Regards
Eagle
Very Nice & Superb solution. Thank you
ReplyDeletehi rajdeep i have done everything as in tutorial when hosted on the iis its showing access is denied exception.
ReplyDeleteHi, I find your tutorials extremely helpful. You cover almost all of the functionality of the GDrive. I would love to see a tutorial how to transfer files between GDrive accounts and How to stream media files and read text and pdf files from the app.
ReplyDeleteThank's again for your tutorials on the subject and hope that you can do something on my request.
upload a tutorial for accessing other user account using oauth 2 authorization ..
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI want to restrict the Google Account where Files get Uploaded ,I just want to host images in google Drive
ReplyDeleteError: redirect_uri_mismatch
ReplyDeleteThe redirect URI in the request, http://localhost:55161/authorize/, does not match the ones authorized for the OAuth client. To update the authorized redirect URIs, visit: https://console.developers.google.com/apis/credentials/oauthclient/949101780019-bn997i03q5sdv6r8ivtlq34nmrvrhpc3.apps.googleusercontent.com?project=949101780019
hello sir,
ReplyDeleteThank you so much for this amazing article
while executing the program am facing the below problem in the page of GoogleDriveFile repository.cs in this statement: (//get file list.
IList files = FileListRequest.Execute().Files;)
Error :
An exception of type 'Google.GoogleApiException' occurred in mscorlib.dll but was not handled in user code
Additional information: Google.Apis.Requests.RequestError
Access Not Configured. Drive API has not been used in project 263396134778 before or it is disabled. Enable it by visiting https://console.developers.google.com/apis/api/drive.googleapis.com/overview?project=263396134778 then retry. If you enabled this API recently, wait a few minutes for the action to propagate to our systems and retry. [403]
Errors [
Message[Access Not Configured. Drive API has not been used in project 263396134778 before or it is disabled. Enable it by visiting https://console.developers.google.com/apis/api/drive.googleapis.com/overview?project=263396134778 then retry. If you enabled this API recently, wait a few minutes for the action to propagate to our systems and retry.] Location[ - ] Reason[accessNotConfigured] Domain[usageLimits]
]