Cloud Storage Access through API - ASP.NET MVC 5 - C# (Part-6)
Google Drive REST API v3-
How to Get Users Permission details of Google Drive items?
2) Google Drive API - File Upload, View, Download & Delete
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
Google Drive REST API v3-
How to Get Users Permission details of Google Drive items?
3) Google Drive API - Create folder, Upload file to folder, Show folder Contain.
4) Google Drive API - Move & Copy files between folders.
5) Google Drive API - Manage Share & Set User Role Permissions.
6) Google Drive API - View Share Users Permission Details.
Source Code: Click to download [30.02 MB]
This tutorial is a continuation of Part-5
In Part-5 of this video series, we have seen "Manage Share with Insert Permission in Google drive using Google drive API v3".
In this tutorial we will learn how to get the permission details of google drive files & folders.
Programmatic each & every contains of Google drive has its own default permission. First time when you are upload a file or create a folder in google drive by default a owner permission will set to this item of owner email address.
One important things you need to remember is that at least one Owner is required otherwise we can't access this file into the google drive.
we are getting this permission details by using IList<Google.Apis.Drive.v3.Data.Permission> collection.
A) Step-1: Add Google Drive API reference in Visual Studio.
First, Goto the Visual Studio 2013 or Higher Version. In my case I am using Visual studio 2017.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following two commands.
PM> Install-Package Google.Apis.Drive.v3
B) Step-2: Get Client Credentials of Google Drive.
First our code getting the user account details from client_secret.json file. Here User account details means client credentials & staring google drive API services.
Source Code:
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
//GetService_v3 method used for Staring Google Drive API version 3 services
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//Create Drive API v3 service.
Google.Apis.Drive.v3.DriveService service =
new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
C) View the contains of Google Drive?
First we need start the google drive API services & after that we are requesting the list of files by using ListRequest class. Here Id, name, size, parents are the requested fields. Execute() method of ListRequest class return those file list as IList<Google.Apis.Drive.v3.Data.File> files collection.
Source Code:
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(id, name, size, parents)";
// List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents
};
FileList.Add(File);
}
}
return FileList;
}
D) How to get Permission details of Google Drive items?
Google drive API return the permission details as IList collection of Permisssion class & we are get this collection by using "permissions" field of FilesResource.GetRequest class object getReq.
Source Code:
public static IList<Google.Apis.Drive.v3.Data.Permission> GetPremissionDetails(string fileId)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.FilesResource.GetRequest getReq = service.Files.Get(fileId);
getReq.Fields = "permissions";
Google.Apis.Drive.v3.Data.File file = getReq.Execute();
return file.Permissions;
}

Let's do it using Visual Studio MVC Application:
First, Goto the Visual Studio 2013 or Higher Version.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following two commands.
PM> Install-Package Google.Apis.Drive.v2
PM> Install-Package Google.Apis.Drive.v3
Here I am using Google Drive API v3,
Step 3 (Model):
First, we need to create one model class under the Model folder of project solution.
Model Class Name: GoogleDriveFiles.cs
Please Copy & Paste this code into this class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public IList<string> Parents { get; set; }
}
}
Step 4 (Repository):
After that, create another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Please Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
namespace GoogleDriveAPI.Models
{
public class GoogleDriveFilesRepository
{
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(GoogleClientSecrets.Load(stream).Secrets, Scopes, "user", CancellationToken.None, new FileDataStore(FilePath, true)).Result;
}
//Create Drive API service.
Google.Apis.Drive.v3.DriveService service = new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(id, name, size, parents)";
// List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents
};
FileList.Add(File);
}
}
return FileList;
}
public static IList<Google.Apis.Drive.v3.Data.Permission> GetPremissionDetails(string fileId)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.FilesResource.GetRequest getReq = service.Files.Get(fileId);
getReq.Fields = "permissions";
Google.Apis.Drive.v3.Data.File file = getReq.Execute();
return file.Permissions;
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
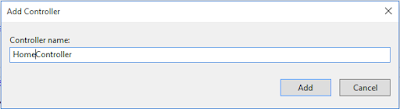
Copy & Paste this code into this controller:
using GoogleDriveAPI.Models;
using System.Collections.Generic;
using System.IO;
using System.Web.Mvc;
namespace GoogleDriveAPI.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpGet]
public JsonResult GetPremissionDetails(string fileId)
{
Dictionary<string, object> jsonResult = new Dictionary<string, object>();
var result = GoogleDriveFilesRepository.GetPremissionDetails(fileId);
jsonResult.Add("Html1", RenderRazorViewToString("~/Views/Home/_GetPermissionUsers.cshtml", result));
return Json(jsonResult, JsonRequestBehavior.AllowGet);
}
public string RenderRazorViewToString(string viewName, object model)
{
if (model != null)
{
ViewData.Model = model;
}
using (var sw = new StringWriter())
{
var viewResult = ViewEngines.Engines.FindPartialView(ControllerContext, viewName);
var viewContext = new ViewContext(ControllerContext, viewResult.View, ViewData, TempData, sw);
viewResult.View.Render(viewContext, sw);
viewResult.ViewEngine.ReleaseView(ControllerContext, viewResult.View);
return sw.GetStringBuilder().ToString();
}
}
}
}
Step 6 (View): We need to create Three view,
First View : GetGoogleDriveFiles.cshtml)
This view for showing the root directory contains Google drive.
Second View: _GetPermissionUsers.cshtml
This view showing permission details of each & every google drive contains.
Third View: _Layout.cshtml (into the Share Folder)
This view maintain the layout of this application.
View1 : Add a view (View Name: GetGoogleDriveFiles.cshtml) of GetGoogleDriveFiles action method.
Copy & Paste this code within this View:
@model IEnumerable<GoogleDriveAPI.Models.GoogleDriveFiles>
@{
ViewBag.Title = "GetGoogleDriveFiles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<!-- The Modal -->
<div id="ModalViewPermission" class="modal">
<!-- Modal content -->
<span id="FileName"></span>
<br />
<br />
<div class="modal-content" id="Render_PremissionDetails">
</div>
</div>
<h2 class="imagetable">Google Drive API v3 - [Root Directory] - Display Share/Permission Details</h2>
<input type="hidden" id="hdFileID" value="" />
<center>
<table class="imagetable">
<tr id="header">
<th>
Share Details
</th>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
Type
</th>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
if (item.Parents[0] == "0ANNR3nwrf6gUUk9PVA")
{
<tr>
<td>
<center><img src="~/Images/Slideshare-Icon.ico" height="40" width="40" data-key="@item.Id" data-name="@item.Name" class="ShareDetails"></center>
</td>
@{
string FileSize = string.Empty;
string Type = string.Empty;
if (@item.Size != null)
{
long? KiloByte = @item.Size;
FileSize = KiloByte + " bytes";
}
else
{
FileSize = "0 bytes";
}
}
<td>
@item.Name
</td>
<td>
@{
if (FileSize == "0 bytes") { Type = "Folder"; }
else { Type = "File"; }
}
@Type
</td>
</tr>
}
}
}
else
{
<tr> <td colspan="7">No Records found</td> </tr>
}
</table>
</center>
<script type="text/javascript">
$(document).on('click', '.ShareDetails', function () {
var FileId = $(this).attr("data-key");
var FileName = $(this).attr("data-name");
$("#FileName").html('<strong>File/Folder Name</strong>: ' + FileName);
$("#hdFileID").val(FileId);
$("#ModalViewPermission").modal('show');
$("#Render_PremissionDetails").html("<center><img src='../../Images/ajax-loading.gif' alt='Loading...' /></center>");
$.ajax({
url: "/Home/GetPremissionDetails",
type: "GET",
data: { fileId: FileId },
contentType: "application/json",
success: function (data) {
$("#Render_PremissionDetails").html(data.Html1);
}
});
});
</script>
View2 : Add another view (View Name: _GetPermissionUsers.cshtml)
Copy & Paste this code within this View:
@model IEnumerable<Google.Apis.Drive.v3.Data.Permission>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<center>
<table class="imagetable" style="width:100%; align-content:center;">
<tr id="header">
<th>
Permission ID
</th>
<th>
Email Address
</th>
<th>
User Type
</th>
<th>
User Role
</th>
<th>
Kind
</th>
</tr>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
<tr id="header">
<td><center>@item.Id</center></td>
<td><center>@item.EmailAddress</center></td>
<td><center>@item.Type</center></td>
<td><center>@item.Role</center></td>
<td><center>@item.Kind</center></td>
</tr>
}
}
else
{
<tr id="header">
<td colspan="6"></td>
</tr>
}
</table>
</center>
View2 : Add another view (View Name: _GetPermissionUsers.cshtml) . create this view into the share folder into view folder.
<!DOCTYPE html>
<html>
<head>
<title>Google Drive Rest API</title>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-modal/0.9.1/jquery.modal.js"></script>
@*<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-modal/0.9.1/jquery.modal.css">*@
<link href="~/Style/Style.css" rel="stylesheet" />
<link href="~/Style/jquery.modal.css" rel="stylesheet" />
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
@Html.ActionLink("Cloud Storage Access through API - ASP.NET MVC 5 - C# - [Part-6]", "GetGoogleDriveFiles", "Home", null, new { @class = "navbar-brand" })
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
</ul>
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
</div>
</body>
</html>
Programmatic each & every contains of Google drive has its own default permission. First time when you are upload a file or create a folder in google drive by default a owner permission will set to this item of owner email address.
One important things you need to remember is that at least one Owner is required otherwise we can't access this file into the google drive.
we are getting this permission details by using IList<Google.Apis.Drive.v3.Data.Permission> collection.
A) Step-1: Add Google Drive API reference in Visual Studio.
First, Goto the Visual Studio 2013 or Higher Version. In my case I am using Visual studio 2017.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following two commands.
PM> Install-Package Google.Apis.Drive.v3
B) Step-2: Get Client Credentials of Google Drive.
First our code getting the user account details from client_secret.json file. Here User account details means client credentials & staring google drive API services.
Source Code:
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
//GetService_v3 method used for Staring Google Drive API version 3 services
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(
GoogleClientSecrets.Load(stream).Secrets,Scopes,
"user",
CancellationToken.None,
new FileDataStore(FilePath, true)).Result;
}
//Create Drive API v3 service.
Google.Apis.Drive.v3.DriveService service =
new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
First we need start the google drive API services & after that we are requesting the list of files by using ListRequest class. Here Id, name, size, parents are the requested fields. Execute() method of ListRequest class return those file list as IList<Google.Apis.Drive.v3.Data.File> files collection.
Source Code:
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(id, name, size, parents)";
// List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents
};
FileList.Add(File);
}
}
return FileList;
}
D) How to get Permission details of Google Drive items?
Google drive API return the permission details as IList collection of Permisssion class & we are get this collection by using "permissions" field of FilesResource.GetRequest class object getReq.
Source Code:
public static IList<Google.Apis.Drive.v3.Data.Permission> GetPremissionDetails(string fileId)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.FilesResource.GetRequest getReq = service.Files.Get(fileId);
getReq.Fields = "permissions";
Google.Apis.Drive.v3.Data.File file = getReq.Execute();
return file.Permissions;
}

Let's do it using Visual Studio MVC Application:
First, Goto the Visual Studio 2013 or Higher Version.
Step 1: Create an Empty MVC Project. Name of the project: GoogleDriveRestAPI.
Step 2: Opening the NuGet Package Manager Console, Select the package source and run this following two commands.
PM> Install-Package Google.Apis.Drive.v2
PM> Install-Package Google.Apis.Drive.v3
Here I am using Google Drive API v3,
First, we need to create one model class under the Model folder of project solution.
Model Class Name: GoogleDriveFiles.cs
Please Copy & Paste this code into this class.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace GoogleDriveRestAPI_v3.Models
{
public class GoogleDriveFiles
{
public string Id { get; set; }
public string Name { get; set; }
public long? Size { get; set; }
public IList<string> Parents { get; set; }
}
}
Step 4 (Repository):
After that, create another class (Name: GoogleDriveFilesRepository.cs) under this same Model folder. Please Copy & Paste this below code.
using Google.Apis.Auth.OAuth2;
using Google.Apis.Services;
using Google.Apis.Util.Store;
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
namespace GoogleDriveAPI.Models
{
public class GoogleDriveFilesRepository
{
public static string[] Scopes = { Google.Apis.Drive.v3.DriveService.Scope.Drive };
public static Google.Apis.Drive.v3.DriveService GetService_v3()
{
UserCredential credential;
using (var stream = new FileStream(@"D:\client_secret.json", FileMode.Open, FileAccess.Read))
{
String FolderPath = @"D:\";
String FilePath = Path.Combine(FolderPath, "DriveServiceCredentials.json");
credential = GoogleWebAuthorizationBroker.AuthorizeAsync(GoogleClientSecrets.Load(stream).Secrets, Scopes, "user", CancellationToken.None, new FileDataStore(FilePath, true)).Result;
}
//Create Drive API service.
Google.Apis.Drive.v3.DriveService service = new Google.Apis.Drive.v3.DriveService(new BaseClientService.Initializer()
{
HttpClientInitializer = credential,
ApplicationName = "GoogleDriveRestAPI-v3",
});
return service;
}
public static List<GoogleDriveFiles> GetDriveFiles()
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
// Define parameters of request.
Google.Apis.Drive.v3.FilesResource.ListRequest FileListRequest = service.Files.List();
FileListRequest.Fields = "nextPageToken, files(id, name, size, parents)";
// List files.
IList<Google.Apis.Drive.v3.Data.File> files = FileListRequest.Execute().Files;
List<GoogleDriveFiles> FileList = new List<GoogleDriveFiles>();
if (files != null && files.Count > 0)
{
foreach (var file in files)
{
GoogleDriveFiles File = new GoogleDriveFiles
{
Id = file.Id,
Name = file.Name,
Size = file.Size,
Parents = file.Parents
};
FileList.Add(File);
}
}
return FileList;
}
public static IList<Google.Apis.Drive.v3.Data.Permission> GetPremissionDetails(string fileId)
{
Google.Apis.Drive.v3.DriveService service = GetService_v3();
Google.Apis.Drive.v3.FilesResource.GetRequest getReq = service.Files.Get(fileId);
getReq.Fields = "permissions";
Google.Apis.Drive.v3.Data.File file = getReq.Execute();
return file.Permissions;
}
}
}
Step 5 (Controller): Add a controller class (HomeController.cs) under this controller folder.
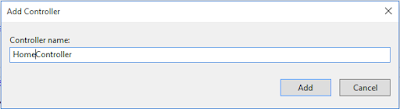
Copy & Paste this code into this controller:
using GoogleDriveAPI.Models;
using System.Collections.Generic;
using System.IO;
using System.Web.Mvc;
namespace GoogleDriveAPI.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public ActionResult GetGoogleDriveFiles()
{
return View(GoogleDriveFilesRepository.GetDriveFiles());
}
[HttpGet]
public JsonResult GetPremissionDetails(string fileId)
{
Dictionary<string, object> jsonResult = new Dictionary<string, object>();
var result = GoogleDriveFilesRepository.GetPremissionDetails(fileId);
jsonResult.Add("Html1", RenderRazorViewToString("~/Views/Home/_GetPermissionUsers.cshtml", result));
return Json(jsonResult, JsonRequestBehavior.AllowGet);
}
public string RenderRazorViewToString(string viewName, object model)
{
if (model != null)
{
ViewData.Model = model;
}
using (var sw = new StringWriter())
{
var viewResult = ViewEngines.Engines.FindPartialView(ControllerContext, viewName);
var viewContext = new ViewContext(ControllerContext, viewResult.View, ViewData, TempData, sw);
viewResult.View.Render(viewContext, sw);
viewResult.ViewEngine.ReleaseView(ControllerContext, viewResult.View);
return sw.GetStringBuilder().ToString();
}
}
}
}
Step 6 (View): We need to create Three view,
First View : GetGoogleDriveFiles.cshtml)
This view for showing the root directory contains Google drive.
Second View: _GetPermissionUsers.cshtml
This view showing permission details of each & every google drive contains.
Third View: _Layout.cshtml (into the Share Folder)
This view maintain the layout of this application.
View1 : Add a view (View Name: GetGoogleDriveFiles.cshtml) of GetGoogleDriveFiles action method.
Copy & Paste this code within this View:
@model IEnumerable<GoogleDriveAPI.Models.GoogleDriveFiles>
@{
ViewBag.Title = "GetGoogleDriveFiles";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<!-- The Modal -->
<div id="ModalViewPermission" class="modal">
<!-- Modal content -->
<span id="FileName"></span>
<br />
<br />
<div class="modal-content" id="Render_PremissionDetails">
</div>
</div>
<h2 class="imagetable">Google Drive API v3 - [Root Directory] - Display Share/Permission Details</h2>
<input type="hidden" id="hdFileID" value="" />
<center>
<table class="imagetable">
<tr id="header">
<th>
Share Details
</th>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
Type
</th>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
if (item.Parents[0] == "0ANNR3nwrf6gUUk9PVA")
{
<tr>
<td>
<center><img src="~/Images/Slideshare-Icon.ico" height="40" width="40" data-key="@item.Id" data-name="@item.Name" class="ShareDetails"></center>
</td>
@{
string FileSize = string.Empty;
string Type = string.Empty;
if (@item.Size != null)
{
long? KiloByte = @item.Size;
FileSize = KiloByte + " bytes";
}
else
{
FileSize = "0 bytes";
}
}
<td>
@item.Name
</td>
<td>
@{
if (FileSize == "0 bytes") { Type = "Folder"; }
else { Type = "File"; }
}
@Type
</td>
</tr>
}
}
}
else
{
<tr> <td colspan="7">No Records found</td> </tr>
}
</table>
</center>
<script type="text/javascript">
$(document).on('click', '.ShareDetails', function () {
var FileId = $(this).attr("data-key");
var FileName = $(this).attr("data-name");
$("#FileName").html('<strong>File/Folder Name</strong>: ' + FileName);
$("#hdFileID").val(FileId);
$("#ModalViewPermission").modal('show');
$("#Render_PremissionDetails").html("<center><img src='../../Images/ajax-loading.gif' alt='Loading...' /></center>");
$.ajax({
url: "/Home/GetPremissionDetails",
type: "GET",
data: { fileId: FileId },
contentType: "application/json",
success: function (data) {
$("#Render_PremissionDetails").html(data.Html1);
}
});
});
</script>
View2 : Add another view (View Name: _GetPermissionUsers.cshtml)
Copy & Paste this code within this View:
@model IEnumerable<Google.Apis.Drive.v3.Data.Permission>
@{
ViewBag.Title = "Google Drive API v3 - ASP.NET MVC 5 [Everyday Be Coding]";
}
<center>
<table class="imagetable" style="width:100%; align-content:center;">
<tr id="header">
<th>
Permission ID
</th>
<th>
Email Address
</th>
<th>
User Type
</th>
<th>
User Role
</th>
<th>
Kind
</th>
</tr>
@if (Model.Count() > 0)
{
foreach (var item in Model)
{
<tr id="header">
<td><center>@item.Id</center></td>
<td><center>@item.EmailAddress</center></td>
<td><center>@item.Type</center></td>
<td><center>@item.Role</center></td>
<td><center>@item.Kind</center></td>
</tr>
}
}
else
{
<tr id="header">
<td colspan="6"></td>
</tr>
}
</table>
</center>
View2 : Add another view (View Name: _GetPermissionUsers.cshtml) . create this view into the share folder into view folder.
<!DOCTYPE html>
<html>
<head>
<title>Google Drive Rest API</title>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-modal/0.9.1/jquery.modal.js"></script>
@*<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-modal/0.9.1/jquery.modal.css">*@
<link href="~/Style/Style.css" rel="stylesheet" />
<link href="~/Style/jquery.modal.css" rel="stylesheet" />
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
@Html.ActionLink("Cloud Storage Access through API - ASP.NET MVC 5 - C# - [Part-6]", "GetGoogleDriveFiles", "Home", null, new { @class = "navbar-brand" })
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
</ul>
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
</div>
</body>
</html>
Thank you for reading this blogs.
Please subscribe my YouTube Channel & don't forget to like and share.
Please subscribe my YouTube Channel & don't forget to like and share.
YouTube : | https://goo.gl/rt4tHH |
Facebook : | https://goo.gl/hgpQsh |
Twitter : | https://goo.gl/nUwGnf |
Comments
Post a Comment