EPPLUS Library - Beginners Guide Part-11(C)
How to Add Multi Style Rich Text in Excel Cell & Comment Using ExcelRichTextCollection class in EPPlus?
Suggested video : EPPLUS Library - Beginners Guide Part-8
Suggested video : EPPLUS Library - Beginners Guide Part-9(A)
Suggested video : EPPLUS Library - Beginners Guide Part-10(B)
Suggested video : EPPLUS Library - Beginners Guide Part-12(D)
How to Add Multi Style Rich Text in Excel Cell & Comment Using ExcelRichTextCollection class in EPPlus?
Suggested video : EPPLUS Library - Beginners Guide Part-8
Suggested video : EPPLUS Library - Beginners Guide Part-9(A)
Suggested video : EPPLUS Library - Beginners Guide Part-10(B)
Suggested video : EPPLUS Library - Beginners Guide Part-12(D)
Hindi Video: Click here
Previous Video: Click here [English]
Previous Video: Click here [Hindi]
Source Code: Click to download [679 KB]
Different types of Format for Excel Comment
- Add. Move, Hide & Remove Comment to an Excel Sheet cell (We already discussed in Part 8)
- Resize/Auto-fit of Comment. (We already discussed in Part 9(A))
- Add Text a Background Color in Comment. (We already discussed in Part 9(A))
- Set Text Alignments in Comment. (We already discussed in Part 10(B))
- Set Font Style in Excel Comment (We already discussed in Part 10(B))
- Multi Style Excel Cell & Comment Text using ExcelRichTextCollection Class. (We already discussed in Part 12(D))
- Set Line or Border Style in a Comment.
How to add Rich Text (Single or Multiple) in Excel Comment using EPPlus?
First, we need to attach one more namespace "OfficeOpenXml.Style".
We have seen in Part-8 of this video series the AddComment() method of ExcelRange class accepts two things as a parameter. 1st parameter is string comment & the second is the string author. Here, the first string parameter is a rich text. Whenever we assign text is Excel comment, rich text is involved automatically. This rich text takes the first position of comment text that means zero index position.
Actually, ExcelComment class object accepts one or more rich text. We can add multiple rich texts by using the RichText property of ExcelComment class. This RichText property is the type of ExcelRichTextCollection class. Indirectly, this collection class is involved by the add() method of this collection class. We can assign each rich text one by one, starting from zero based index positions.
Please see this below code.
- using(ExcelRange Rng = wsSheet1.Cells["B5"]) {
- Rng.Value = "Add Multiple Rich Text in Excel Comment by Index Position.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Rich Text [Index 0]\n", "Rajdip");
- //We can Add multiple Rich Text in ExcelComment object
- cmd.RichText.Add("Rich Text [Index 1]\n");
- cmd.RichText.Add("Rich Text [Index 2]\n");
- cmd.RichText.Add("Rich Text [Index 3]");
- cmd.Visible = true;
- }
Please see this below code.
- using(ExcelRange Rng = wsSheet1.Cells["B10"]) {
- Rng.Value = "Add Multi Style Rich Text in Excel Comment.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Everyday Be Coding ", "Rajdip");
- cmd.Font.Bold = true;
- cmd.Font.UnderLine = true;
- cmd.Font.Italic = true;
- cmd.Font.Color = Color.Red;
- cmd.Font.FontName = "Arial Rounded MT Bold";
- //RichText at Position 1
- ExcelRichText RichText1 = cmd.RichText.Add("is my ");
- RichText1.Bold = true;
- RichText1.Italic = false;
- RichText1.Color = Color.Green;
- RichText1.FontName = "Arial Narrow";
- //RichText at Position 2
- ExcelRichText RichText2 = cmd.RichText.Add("YouTube ");
- RichText2.Bold = true;
- RichText2.Italic = false;
- RichText2.Color = Color.Blue;
- RichText2.FontName = "Arial";
- //RichText at Position 3
- ExcelRichText RichText3 = cmd.RichText.Add("Channel.");
- RichText3.Bold = true;
- RichText3.Italic = false;
- RichText3.Color = Color.Brown;
- RichText3.FontName = "Arial Black";
- cmd.Visible = true;
- }
How Remove Rich Text in Excel Comment using EPPlus?
We can also remove ExcelRichText object using RemoveAt(int Index) method (by index position) of ExcelRichTextCollection class.
In this code, RichText is the property of ExcelComment class & RichText property is the type of ExcelRichTextCollection class.
Please see this below code.
- using(ExcelRange Rng = wsSheet1.Cells["B15"]) {
- Rng.Value = "Remove Rich Text in Excel Comment by Index Position.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Rich Text [Index 0]\n", "Rajdip");
- cmd.RichText.Add("Rich Text [Index 1]\n");
- cmd.RichText.Add("Rich Text [Index 2]\n");
- cmd.RichText.Add("Rich Text [Index 3]");
- //Remove Rich Text at Second position.
- cmd.RichText.RemoveAt(2);
- //string count = cmd.RichText.Count.ToString();
- cmd.Visible = true;
- }
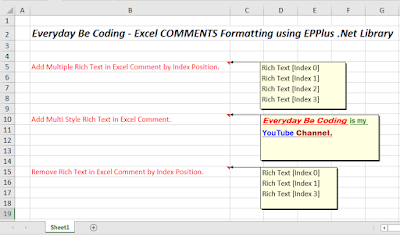
Full Source code
- using OfficeOpenXml;
- using System.IO;
- using System.Drawing;
- using OfficeOpenXml.Style;
- namespace EpplusDemo {
- class Program {
- static void Main(string[] args) {
- //Code download from: https://everyday-be-coding.blogspot.in/p/epplus-library-part-11.html
- //Author: Rajdip Sarkar.
- //Date : 3rd July 2017.
- //My YouTube Channel Link : https://www.youtube.com/channel/UCpGuQx5rDbWnc7i_qKDTRSQ
- ExcelPackage ExcelPkg = new ExcelPackage();
- ExcelWorksheet wsSheet1 = ExcelPkg.Workbook.Worksheets.Add("Sheet1");
- using(ExcelRange Rng = wsSheet1.Cells[2, 2, 2, 2]) {
- Rng.Value = "Everyday Be Coding - Excel COMMENTS Formatting using EPPlus .Net Library";
- Rng.Style.Font.Size = 16;
- Rng.Style.Font.Bold = true;
- Rng.Style.Font.Italic = true;
- }
- //Add Multiple Rich Text Box in Excel Comment by Index Position.
- using(ExcelRange Rng = wsSheet1.Cells["B5"]) {
- Rng.Value = "Add Multiple Rich Text in Excel Comment by Index Position.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Rich Text [Index 0]\n", "Rajdip");
- //We can Add multiple Rich Text in ExcelComment object
- cmd.RichText.Add("Rich Text [Index 1]\n");
- cmd.RichText.Add("Rich Text [Index 2]\n");
- cmd.RichText.Add("Rich Text [Index 3]");
- cmd.Visible = true;
- }
- //How to Add Multi Style Rich Text in Excel Comment
- using(ExcelRange Rng = wsSheet1.Cells["B10"]) {
- Rng.Value = "Add Multi Style Rich Text in Excel Comment.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Everyday Be Coding ", "Rajdip");
- cmd.Font.Bold = true;
- cmd.Font.UnderLine = true;
- cmd.Font.Italic = true;
- cmd.Font.Color = Color.Red;
- cmd.Font.FontName = "Arial Rounded MT Bold";
- //RichText at Position 1
- ExcelRichText RichText1 = cmd.RichText.Add("is my ");
- RichText1.Bold = true;
- RichText1.Italic = false;
- RichText1.Color = Color.Green;
- RichText1.FontName = "Arial Narrow";
- //RichText at Position 2
- ExcelRichText RichText2 = cmd.RichText.Add("YouTube ");
- RichText2.Bold = true;
- RichText2.Italic = false;
- RichText2.Color = Color.Blue;
- RichText2.FontName = "Arial";
- //RichText at Position 3
- ExcelRichText RichText3 = cmd.RichText.Add("Channel.");
- RichText3.Bold = true;
- RichText3.Italic = false;
- RichText3.Color = Color.Brown;
- RichText3.FontName = "Arial Black";
- cmd.Visible = true;
- }
- //Remove Rich Text in Excel Comment by Index Position.
- using(ExcelRange Rng = wsSheet1.Cells["B15"]) {
- Rng.Value = "Remove Rich Text in Excel Comment by Index Position.";
- Rng.Style.Font.Color.SetColor(Color.Red);
- ExcelComment cmd = Rng.AddComment("Rich Text [Index 0]\n", "Rajdip");
- cmd.RichText.Add("Rich Text [Index 1]\n");
- cmd.RichText.Add("Rich Text [Index 2]\n");
- cmd.RichText.Add("Rich Text [Index 3]");
- //Remove Rich Text at Second position.
- cmd.RichText.RemoveAt(2);
- //string count = cmd.RichText.Count.ToString();
- cmd.Visible = true;
- }
- wsSheet1.Cells[wsSheet1.Dimension.Address].AutoFitColumns();
- ExcelPkg.SaveAs(new FileInfo(@ "D:\FormatExcelComments.xlsx"));
- }
- }
- }
Now, build & execute this code. File is (FormatExcelComments.xlsx) stored on D: drive of computer.
Please subscribe my YouTube Channel & don't forget to like and share.
Please subscribe my YouTube Channel & don't forget to like and share.
YouTube : | https://goo.gl/rt4tHH |
Facebook : | https://goo.gl/m2skDb |
Twitter : | https://goo.gl/nUwGnf |
Comments
Post a Comment